First of all, in C++ your implementation should be separate from the declaration unless you are using templates which you are not. This is not Java.
Second, your sample code is missing a closing brace. I have submitted an edit to add it and improve your code formatting.
That aside, the following implementation works for me:
Item.h
class Item
{
private:
std::string name;
public:
void set_data();
void printName();
};
Item.cpp
void Item::set_data()
{
std::cout << "Type name and hit return: " << std::endl;
getline(std::cin, name);
}
void Item::printName()
{
std::cout << "Name is : " << name << std::endl;
}
main.cpp
// Entry point
int main(int argc, char** argv)
{
// Yes I thought I would mix things up and use new instead of Item i; So shoot me.
Item * i = new Item();
i->set_data();
i->printName();
i->set_data();
i->printName();
delete i;
return 0;
}
The application will wait at both calls to set_data()
for me to type something in and hit return. Then it continues as normal. I added a text prompt so it is less confusing to see in the console. I get this:
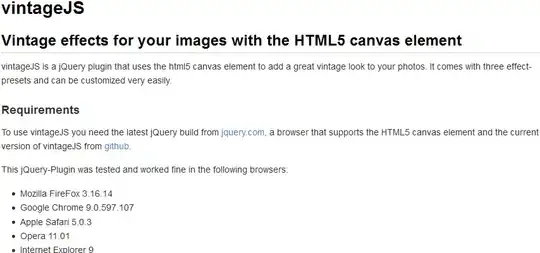
Does this in some way answer your question? If you are doing something else in main()
then try stripping it out back to just this simple action then add the other stuff back in until you find the bit that introduces the problem.
UPDATE:
As prompted by the comments below, if you put std::cin >>
before another call to getline()
it will read the first word from the stream and leave the rest of your string and the \n
character in there which getline()
uses for its delimiter. So next time you call getline()
it will automatically extract the rest of the string from the stream without requesting user input. I guess this is probably what you are seeing.