You probably should read more about such type as BLOB
.
Your question combines two themes:
- How to upload media using JSP
- How to store image in DB
Both question were already several times answered on this site but below you can find some instructions in one place. Because you didn't provide information about which DB do you use I will explain how to make this working in oracle.
1.First you need to create new user in oracle. Below you can find sql script for creation of TEST
user.
CREATE USER TEST IDENTIFIED BY TEST DEFAULT TABLESPACE USERS TEMPORARY TABLESPACE TEMP PROFILE DEFAULT ACCOUNT UNLOCK;
GRANT CONNECT, RESOURCE, IMP_FULL_DATABASE, CREATE VIEW, UNLIMITED TABLESPACE, CREATE JOB TO TEST;
2.Then you need to create a table with column image
which will store your image
create table test.image_storage (image blob);
3.Create your JSP and create form for your image upload:
<form action="upload" method="post" enctype="multipart/form-data">
<input type="text" name="description" />
<input type="file" name="file" />
<input type="submit" />
</form>
4.Register your servlet:
import javax.servlet.ServletException;
import javax.servlet.annotation.MultipartConfig;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.Part;
import java.io.IOException;
import java.io.InputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
@WebServlet("/upload")
@MultipartConfig
public class UploadServlet extends HttpServlet {
public static final String QUERY = "INSERT INTO IMAGE_STORAGE (IMAGE) VALUES (?)";
public static final String CONNECTION_URL = "jdbc:oracle:thin:@localhost:1522:orcl";
public static final String USER_NAME = "TEST";
public static final String USER_PWD = "TEST";
public static final String FILE_PARAMETER = "file";
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
Part filePart = request.getPart(FILE_PARAMETER);
InputStream fileContent = filePart.getInputStream();
try {
Class.forName("oracle.jdbc.driver.OracleDriver");
} catch (ClassNotFoundException e) {
System.out.println("Oracle driver is absent?");
e.printStackTrace();
}
Connection connection = null;
try {
connection = DriverManager.getConnection(
CONNECTION_URL, USER_NAME, USER_PWD);
PreparedStatement statement = null;
try {
statement = connection.prepareStatement(QUERY);
statement.setBlob(1, fileContent);
statement.execute();
} catch (SQLException e) {
System.out.println("State cannot be executed!");
e.printStackTrace();
return;
} finally {
statement.close();
}
} catch (SQLException e) {
System.out.println("Connection Failed!");
e.printStackTrace();
return;
} finally {
try {
connection.close();
} catch (SQLException e) {
System.out.println("Connection cannot be closed?");
e.printStackTrace();
}
}
}
}
Once you will upload an image you will see a new entry in your DB:
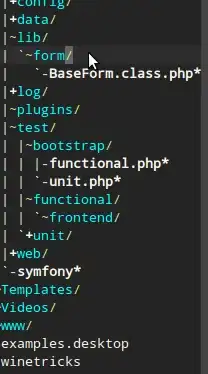
Hope this will help you to understand this topic.