I think you want an Android app able to do this. First of all you have Android Studio installed so in Android Studio click on File
-> New
-> New Project...
and let's create the project as shown here. Your project location will be different and it's ok but if you don't want to have troubles later please leave the same package name
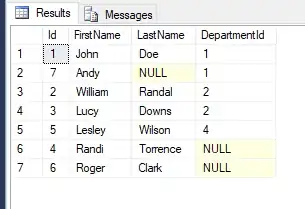
then leave the default in the next screen and finally choose the Empty Activity:
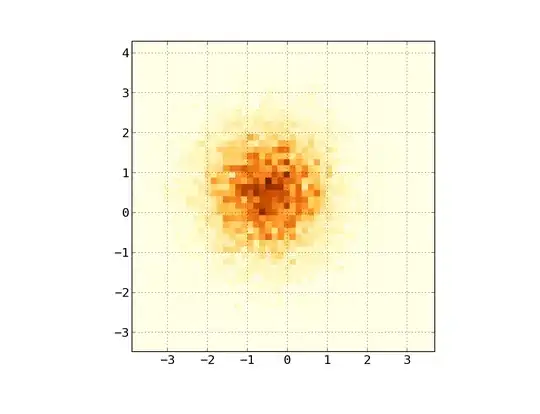
then Next and in the next screen leave the default settings and click Finish
Now in order to build this app we have to modify the files that I'm gonna show you
The first file is essentially the layout of your app. To be fully precise hardcoding 16dp
as I did here is not the best practice but for the moment it will be ok
In the left menu go to app/res/layout
and double click activity_main.xml
You can see both the graphics and a code depending on if you are respectively clicking on Design
or Text
tab. You need to select this last one and copy and paste this code:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="16dp"
android:paddingLeft="16dp"
android:paddingRight="16dp"
android:paddingTop="16dp"
tools:context="com.stackoverflow.mario.MainActivity" >
<TextView
android:id="@+id/textView1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_centerHorizontal="true"
android:layout_marginTop="97dp"
android:text="Ratio"
android:textAppearance="?android:attr/textAppearanceMedium"
android:textSize="@dimen/abc_action_bar_default_height_material" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentLeft="true"
android:layout_below="@+id/textView1"
android:layout_marginLeft="20dp"
android:layout_marginTop="43dp"
android:text="Number One"
android:textAppearance="?android:attr/textAppearanceMedium" />
<EditText
android:id="@+id/txtNumber1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignBottom="@+id/textView2"
android:layout_alignRight="@+id/textView1"
android:ems="2"
android:singleLine="true"
android:inputType="numberDecimal" >
<requestFocus />
</EditText>
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/textView2"
android:layout_below="@+id/textView2"
android:layout_marginTop="47dp"
android:text="Number Two"
android:textAppearance="?android:attr/textAppearanceMedium" />
<Button
android:id="@+id/btnAdd"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignLeft="@+id/textView3"
android:layout_alignRight="@+id/textView3"
android:layout_below="@+id/textView3"
android:layout_marginTop="46dp"
android:onClick="onClick"
android:text="Divide" />
<EditText
android:id="@+id/txtNumber2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@+id/btnAdd"
android:layout_alignLeft="@+id/txtNumber1"
android:ems="2"
android:singleLine="true"
android:inputType="numberDecimal" />
<TextView
android:id="@+id/txtResult"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignRight="@+id/txtNumber2"
android:layout_alignTop="@+id/btnAdd"
android:textAppearance="?android:attr/textAppearanceMedium" />
</RelativeLayout>
The second file is essentially the engine of your app. Go to app/java/com/stackoverflow/mario
and click on MainActivity
[.java]. Copy and paste the following code:
package com.stackoverflow.mario;
import android.os.Bundle;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
EditText firstNumber;
EditText secondNumber;
TextView addResult;
Button btnAdd;
double num1,num2,ratio;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
firstNumber = (EditText)findViewById(R.id.txtNumber1);
secondNumber = (EditText)findViewById(R.id.txtNumber2);
addResult = (TextView)findViewById(R.id.txtResult);
btnAdd = (Button)findViewById(R.id.btnAdd);
btnAdd.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
num1 = Double.parseDouble(firstNumber.getText().toString());
num2 = Double.parseDouble(secondNumber.getText().toString());
ratio = num1 / num2;
addResult.setText(Double.toString(ratio));
}
});
}
}
Now if you build [in Android Studio: Build
-> Rebuild Project
] and run [in Android Studio: Run
-> Run App
] this app you will see a screen like this:
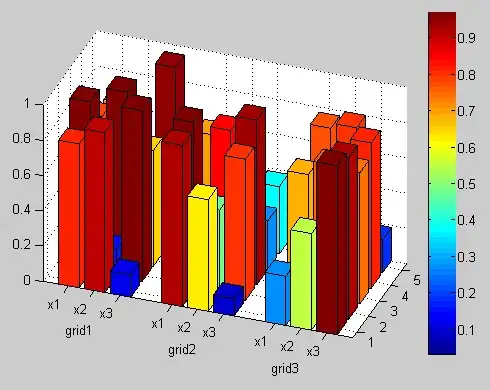
You can run the app creating a virtual device [the previous screenshot is from the Android Emulator and you can create one virtual device clicking the button shown in this screenshot]
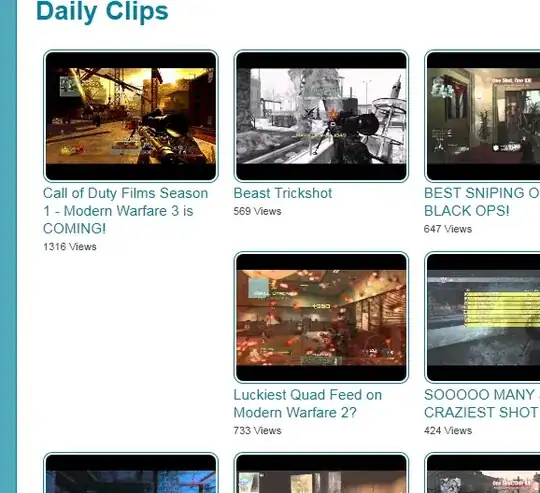
or if you have your phone you can enable the USB debugging, connect the USB to the PC, select your device from the window showing the devices and then clicking OK
credits for a similar sample to the author of this post