I'm assuming that your JSP file looks like this:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<title>$Title$</title>
</head>
<body>
<%
String name = request.getParameter("realName");
%>
Here's the param "realName": <%=name%>
</body>
</html>
And that it looks like this, in your IntelliJ:

If that's the case, I'm almost sure you're missing the servlet-api.jar
file in your classpath.
Here's one of the ways to add it on IntelliJ:
- Right-click on your project and select Open Module Settings:
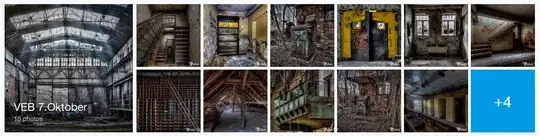
- Make sure that you're on the Modules section, Dependencies tab, click on the "+" button at the bottom, and select 1 JARs or directories...:

- Select the file
servlet-api.jar
from the folder lib
at (THIS IS IMPORTANT:) the container where you're deploying your application (in my case, apache-tomcat-8.5.31):
- Then click on the "Ok" button. Your program now should look like this:
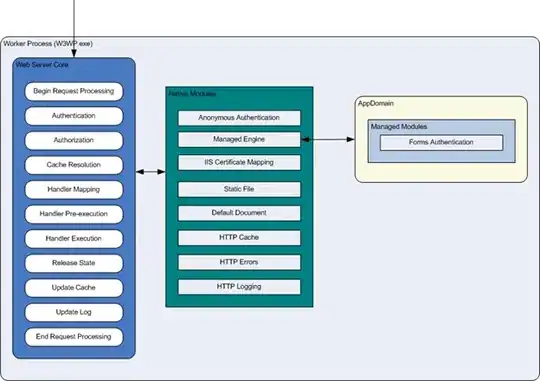
You're good to go!
I hope it helps.
Note: I know that sometimes you cannot avoid to use scriptlets, especially when you're working on legacy codes, as I did for a while. Even though, please also pay attention to the other answers here about using scriptlets. There are several other options available.