You can take a look at Flex Docs!
Adding flexDirection to a component's style determines the primary axis of its layout.
and then:
Adding alignItems to a component's style determines the alignment of children along the secondary axis (if the primary axis is row, then the secondary is column, and vice versa).
So your desired code will be:
import React, { Component } from 'react';
import { AppRegistry, View } from 'react-native';
export default class AlignItemsBasics extends Component {
render() {
return (
<View style={{
flex: 1,
flexDirection: 'column',
justifyContent: 'center',
alignItems: 'center',
}}>
<View style={{width: 50, height: 50, backgroundColor: 'powderblue'}} />
<View style={{width: 50, height: 50, backgroundColor: 'skyblue'}} />
<View style={{width: 50, height: 50, backgroundColor: 'steelblue'}} />
</View>
);
}
};
// skip this line if using Create React Native App
AppRegistry.registerComponent('AwesomeProject', () => AlignItemsBasics);
UPDATE
If you mean something like this image:
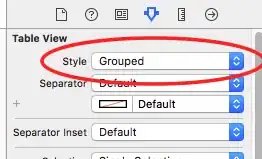
Then I'll suggest you this:
import React, { Component } from "react";
import { View, StyleSheet } from "react-native";
class Playground extends Component {
render() {
return (
<View style={styles.container}>
<View style={styles.boxes} />
<View style={styles.boxes} />
<View
style={[
styles.boxes,
{
backgroundColor: "crimson",
position: "absolute",
right: 0
}
]}
/>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
flexDirection: "row",
justifyContent: "flex-start",
alignItems: "center"
},
boxes: {
width: 50,
height: 50,
marginLeft: 1, // to separate each box!
backgroundColor: "steelblue"
}
});
export default Playground;
As far as i know with these props, it's the best way!