Here is a simple/low-frills method. It doesn't have any external dependencies as you mentioned.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Use PHP in JavaScript</title>
</head>
<body>
<?php
// assuming your database call returns a valid array like this
$things = array("banner_1.jpg", "banner_2.jpg", "banner_3.jpg", "banner_4.jpg");
// the built-in `json_encode` will make JavaScript like it
$things_json = json_encode($things);
?>
<div class="etc">
<!--this is were the database items will go-->
<ul id="things"></ul>
</div>
<script>
// echo out json-ified PHP directly into the script tag
var things = <?php echo $things_json ?>;
// loop through the json and append list items to our target element
for(var i = 0; i < things.length; i++) {
document.querySelector('body').innerHTML += '<li>' + things[i] + '</li>';
}
</script>
</body>
</html>
The main tricks here are:
- use
json_encode($your_array_here)
, which is built in to php
echo
the json directly into a script tag in the body.
You can even work with the new things
variable from an external script assuming you keep it in the global scope and have your external js run on DOMContentLoaded
or similar.
Here are some images of the code working:
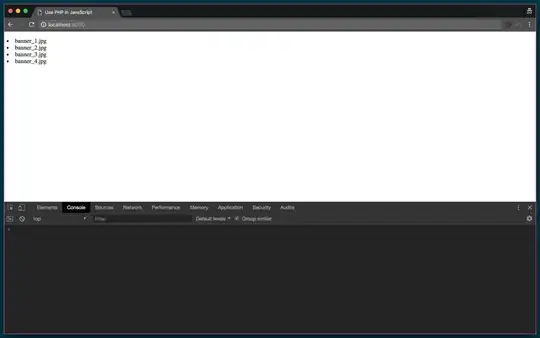
Note that json_encode()
will only accept one variable at a time. If you need to pass multiple variables down into the script, you can build up an array (map) in php with array functions, or just json_encode()
each variable separately and make multiple echo calls inside the script tag.
<?php
// assuming your database call returns a valid array like this
$things = array("banner_1.jpg", "banner_2.jpg", "banner_3.jpg", "banner_4.jpg");
$more_things = array("banner_5.jpg", "banner_6.jpg");
$both_things = array_merge($things, $more_things);
$both_things_json = json_encode($both_things);
?>
<script>
// echo out json-ified PHP directly into the script tag
var bothThings = <?php echo $both_things_json ?>;
</script>