How about this?
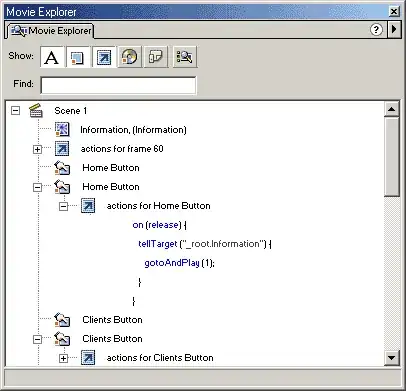
Based on Louys Patrice Bessette's answer I drew up a quick solution that should be more native to the picker and works for also allowing specific hour ranges (e.g. opening hours, etc.) - I believe this may be helpful to some of you, since it doesn't require to re-chose or any timeouts for displaying or anything "hacked"
There's only 1 change required in the *-clockpicker.js inside function mousedown(e, space)
around line 243, just add
// When clicking on disabled field -> do nothing
if ($(e.target).closest('.clockpicker-tick-disabled').length === 1) {
e.preventDefault();
return false;
}
in the very beginning of the function.
Then similar to the answer given add your clock picker control
<div id="time_picker" class="input-group clockpicker">
<input placeholder="Time" type="text" class="form-control">
<span class="input-group-addon">
<span class="fa fa-clock-o fa-2"></span>
</span>
</div>
define your valid (e.g. hour) range
var hour_min = 10;
var hour_max = 17;
var ticksDisabled = false;
and bind the mouseover function to figure out whether this specific (e.g. hour) is available or not
// Handlers to toggle the "hiddenTicksDisabled"
$(document).on("mouseenter",".clockpicker-hours>.clockpicker-tick-disabled",function(e){
ticksDisabled = true;
});
$(document).on("mouseenter",".clockpicker-hours>.clockpicker-tick",function(e){
ticksDisabled = false;
});
Then initialize the picker and change the class for all items that are not in the valid range (update the rule to your liking)
$('#time_picker').clockpicker({
autoclose: true,
donetext: "Done",
afterShow: function() { // Disable all hours out of range
$(".clockpicker-hours").find(".clockpicker-tick").filter(function(index,element){
return !(parseInt($(element).html()) >= hour_min && parseInt($(element).html()) <= hour_max);
}).removeClass('clockpicker-tick').addClass('clockpicker-tick-disabled');
},
});
In order to have the "grayed-out" hours outside valid range and the "not-allowed" mouse pointer on the plate, I added some styles
.clockpicker-tick-disabled {
border-radius: 50%;
color: rgb(192, 192, 192);
line-height: 26px;
text-align: center;
width: 26px;
height: 26px;
position: absolute;
cursor: not-allowed;
}
Have fun!