These are the main functions.
dailysheets.gs:
function archiveDaily() {
const mshsr=4;
const ss=SpreadsheetApp.getActive();
const msh=ss.getSheetByName('Master');
const dss=SpreadsheetApp.openById(getGlobal('MasterSheetsLogId'));//open master archive
const ts=Utilities.formatDate(new Date(), Session.getScriptTimeZone(), "MM/dd/yy HH:mm:ss")
msh.copyTo(dss).setName(ts);//copies current master sheet to master archive not sure if you would want this but I would
if(msh.getLastRow()-mshsr+1>0) {
msh.getRange(mshsr,1,msh.getLastRow()-mshsr+1,msh.getLastColumn()).clearContent();
}
const nA=getGlobal('DailySheetNames').split(',');//sheet name store in Globals sheet as a hash table
const dt=new Date();
const td=new Date(dt.getFullYear(),dt.getMonth(),dt.getDate()).valueOf();//start of day
const to=new Date(dt.getFullYear(),dt.getMonth(),dt.getDate()+1).valueOf();//end of day
const dshsr=4;
nA.forEach(function(name,i){
let dsh=ss.getSheetByName(name);
let drg=dsh.getRange(dshsr,1,dsh.getLastRow()-dshsr+1,dsh.getLastColumn());
let v=drg.getDisplayValues();
let mv=[[dsh.getName(),'','','','','','','','','','','','','','','','']];//Display Sheetname on top of every group
v.forEach(function(r,i){
let idt=new Date(r[0]).valueOf();
//Select rows that fall between midnights
if(idt>td && idt<to) {
mv.push(r);
}
});
msh.getRange(msh.getLastRow()+1,1,mv.length,mv[0].length).setValues(mv);//Put each sheets data into master sheet
});
}
function createDailyTrigger() {
var ss=SpreadsheetApp.getActive();
//This line keeps you from creating more than one trigger
if(!isTrigger('archiveDaily')) {
ScriptApp.newTrigger('archiveDaily').timeBased().everyDays(1).atHour(23).create();
}
}
These files are just some support files that I use a lot and I used a few things from them in this project.
triggers.gs:
//Filename: triggers.gs
function deleteTrigger(triggerName){
var triggers=ScriptApp.getProjectTriggers();
for (var i=0;i<triggers.length;i++){
if (triggerName==triggers[i].getHandlerFunction()){
ScriptApp.deleteTrigger(triggers[i]);
}
}
}
function isTrigger(funcName){
var r=false;
if(funcName){
var allTriggers=ScriptApp.getProjectTriggers();
for(var i=0;i<allTriggers.length;i++){
if(funcName==allTriggers[i].getHandlerFunction()){
r=true;
break;
}
}
}
return r;
}
function deleteAllTriggers(){
var triggers=ScriptApp.getProjectTriggers();
for (var i=0;i<triggers.length; i++){
ScriptApp.deleteTrigger(triggers[i]);
}
}
function displayProjectTriggers() {
var tA=ScriptApp.getProjectTriggers();
var html="<style>th,td{border:1px solid black;padding:2px;margin:2px;}</style><table><tr><th>Handler Function</th><th>Trigger Type</th><th>Unique ID</th><td> </td></tr>";
for(var i=0;i<tA.length;i++) {
html+=Utilities.formatString('<tr><td>%s</td><td>%s</td><td>%s</td><td><input type="button" value="Delete" onClick="google.script.run.withSuccessHandler(function(){google.script.run.displayProjectTriggers();}).deleteTrigger(\'%s\');" /></td></tr>',tA[i].getHandlerFunction(),tA[i].getEventType(),tA[i].getUniqueId(),tA[i].getHandlerFunction());
}
html+='</table><br /><input type="button" value="Close" onClick="google.script.host.close();" />';
var userInterface=HtmlService.createHtmlOutput(html).setWidth(800);
SpreadsheetApp.getUi().showModelessDialog(userInterface, 'Project Triggers');
}
globals.gs:
I create a sheet named Globals where I store some sheet names and the id of the spreadsheet where I copy the master sheet to just before clearing the contents.
function getGlobals(){
var ss=SpreadsheetApp.getActive();
var sh=ss.getSheetByName('Globals');
var rg=sh.getRange(1,1,sh.getLastRow(),2);
var vA=rg.getValues();
var g={};
for(var i=0;i<vA.length;i++){
g[vA[i][0]]=vA[i][1];
}
return g;
}
function setGlobals(dfltObj){
var dfltH=Object.keys(dfltObj).length;
if(dfltObj){
var ss=SpreadsheetApp.getActive();
var sh=ss.getSheetByName('Globals');
var rg=sh.getRange(1,1,dfltH,2);
var vA=rg.getValues();
for(var i=0;i<dfltH;i++){
vA[i][1]=dfltObj[vA[i][0]];
}
rg.setValues(vA);
}
}
function getGlobal(name){
return getGlobals()[name];
}
function setGlobal(name,value){
var curObj=getGlobals();
if(!curObj.hasOwnProperty(name)) {
var ss=SpreadsheetApp.getActive();
var sh=ss.getSheetByName('Globals');
sh.appendRow([name,value])
}else{
curObj[name]=value;
setGlobals(curObj);
}
}
function cleanGlobals() {
var ss=SpreadsheetApp.getActive();
var sh=ss.getSheetByName('Globals');
if(sh.getLastColumn()>2) {
sh.getRange(1,3,sh.getLastRow(),sh.getLastColumn()-2).clearContent();
}
var rg=sh.getRange(1,1,sh.getLastRow(),2);
var vA=rg.getValues();
for(var i=0;i<vA.length;i++) {
if(!vA[i][0] || !vA[i][1]) {
var userInterface=HtmlService.createHtmlOutput('Globals Sheet Requires Maintenance...Do it know.' + ' Check Row ' + Number(i + 1));
SpreadsheetApp.getUi().showModelessDialog(userInterface, 'Global Maintenance Required');
break;
}
}
}
Codes.gs:
I have a menu setup here.
function onOpen(){
makeMenu();
}
function makeMenu(){
SpreadsheetApp.getUi().createMenu('My Tools')
.addItem('Archive','archiveDaily')
.addItem('Display Project Triggers', 'displayProjectTriggers')
.addItem('Create Daily Trigger', 'createDailyTrigger')
.addToUi();
}
Image of one of the sheets:
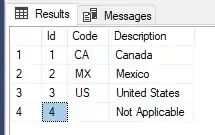
csv for one of the data sheets:
1234567891011121314151617
012345678910111213141516
HDR1HDR2HDR3HDR4HDR5HDR6HDR7HDR8HDR9HDR10HDR11HDR12HDR13HDR14HDR15HDR16HDR17
Mon Feb 10 2020 06:00:00 GMT-0700 (Mountain Standard Time)12345678910111213141516
Mon Feb 10 2020 06:20:00 GMT-0700 (Mountain Standard Time)234567891011121314151617
Mon Feb 10 2020 06:40:00 GMT-0700 (Mountain Standard Time)3456789101112131415161718
Mon Feb 10 2020 07:00:00 GMT-0700 (Mountain Standard Time)45678910111213141516171819
Mon Feb 10 2020 07:20:00 GMT-0700 (Mountain Standard Time)567891011121314151617181920
Mon Feb 10 2020 07:40:00 GMT-0700 (Mountain Standard Time)6789101112131415161718192021
Mon Feb 10 2020 08:00:00 GMT-0700 (Mountain Standard Time)78910111213141516171819202122
Mon Feb 10 2020 08:20:00 GMT-0700 (Mountain Standard Time)891011121314151617181920212223
Mon Feb 10 2020 08:40:00 GMT-0700 (Mountain Standard Time)9101112131415161718192021222324
Mon Feb 10 2020 09:00:00 GMT-0700 (Mountain Standard Time)10111213141516171819202122232425
Mon Feb 10 2020 09:20:00 GMT-0700 (Mountain Standard Time)11121314151617181920212223242526
Mon Feb 10 2020 09:40:00 GMT-0700 (Mountain Standard Time)12131415161718192021222324252627
Mon Feb 10 2020 10:00:00 GMT-0700 (Mountain Standard Time)13141516171819202122232425262728
Mon Feb 10 2020 10:20:00 GMT-0700 (Mountain Standard Time)14151617181920212223242526272829
Mon Feb 10 2020 10:40:00 GMT-0700 (Mountain Standard Time)15161718192021222324252627282930
Mon Feb 10 2020 11:00:00 GMT-0700 (Mountain Standard Time)16171819202122232425262728293031
Mon Feb 10 2020 11:20:00 GMT-0700 (Mountain Standard Time)17181920212223242526272829303132
Mon Feb 10 2020 11:40:00 GMT-0700 (Mountain Standard Time)18192021222324252627282930313233
Image of the master sheet:

csv for Master Sheet:
1234567891011121314151617
012345678910111213141516
HDR1HDR2HDR3HDR4HDR5HDR6HDR7HDR8HDR9HDR10HDR11HDR12HDR13HDR14HDR15HDR16HDR17
Adam
Mon Feb 10 2020 06:00:00 GMT-0700 (Mountain Standard Time)12345678910111213141516
Mon Feb 10 2020 06:20:00 GMT-0700 (Mountain Standard Time)234567891011121314151617
Mon Feb 10 2020 06:40:00 GMT-0700 (Mountain Standard Time)3456789101112131415161718
Mon Feb 10 2020 07:00:00 GMT-0700 (Mountain Standard Time)45678910111213141516171819
Mon Feb 10 2020 07:20:00 GMT-0700 (Mountain Standard Time)567891011121314151617181920
Mon Feb 10 2020 07:40:00 GMT-0700 (Mountain Standard Time)6789101112131415161718192021
Mon Feb 10 2020 08:00:00 GMT-0700 (Mountain Standard Time)78910111213141516171819202122
Mon Feb 10 2020 08:20:00 GMT-0700 (Mountain Standard Time)891011121314151617181920212223
Mon Feb 10 2020 08:40:00 GMT-0700 (Mountain Standard Time)9101112131415161718192021222324
Mon Feb 10 2020 09:00:00 GMT-0700 (Mountain Standard Time)10111213141516171819202122232425
Mon Feb 10 2020 09:20:00 GMT-0700 (Mountain Standard Time)11121314151617181920212223242526
Mon Feb 10 2020 09:40:00 GMT-0700 (Mountain Standard Time)12131415161718192021222324252627
Mon Feb 10 2020 10:00:00 GMT-0700 (Mountain Standard Time)13141516171819202122232425262728
Mon Feb 10 2020 10:20:00 GMT-0700 (Mountain Standard Time)14151617181920212223242526272829
Mon Feb 10 2020 10:40:00 GMT-0700 (Mountain Standard Time)15161718192021222324252627282930
Mon Feb 10 2020 11:00:00 GMT-0700 (Mountain Standard Time)16171819202122232425262728293031
Mon Feb 10 2020 11:20:00 GMT-0700 (Mountain Standard Time)17181920212223242526272829303132
Mon Feb 10 2020 11:40:00 GMT-0700 (Mountain Standard Time)18192021222324252627282930313233
Bill
Mon Feb 10 2020 06:00:00 GMT-0700 (Mountain Standard Time)12345678910111213141516
Mon Feb 10 2020 06:20:00 GMT-0700 (Mountain Standard Time)234567891011121314151617
Mon Feb 10 2020 06:40:00 GMT-0700 (Mountain Standard Time)3456789101112131415161718
Mon Feb 10 2020 07:00:00 GMT-0700 (Mountain Standard Time)45678910111213141516171819
Mon Feb 10 2020 07:20:00 GMT-0700 (Mountain Standard Time)567891011121314151617181920
Mon Feb 10 2020 07:40:00 GMT-0700 (Mountain Standard Time)6789101112131415161718192021
Mon Feb 10 2020 08:00:00 GMT-0700 (Mountain Standard Time)78910111213141516171819202122
Mon Feb 10 2020 08:20:00 GMT-0700 (Mountain Standard Time)891011121314151617181920212223
Mon Feb 10 2020 08:40:00 GMT-0700 (Mountain Standard Time)9101112131415161718192021222324
Mon Feb 10 2020 09:00:00 GMT-0700 (Mountain Standard Time)10111213141516171819202122232425
Mon Feb 10 2020 09:20:00 GMT-0700 (Mountain Standard Time)11121314151617181920212223242526
Mon Feb 10 2020 09:40:00 GMT-0700 (Mountain Standard Time)12131415161718192021222324252627
Mon Feb 10 2020 10:00:00 GMT-0700 (Mountain Standard Time)13141516171819202122232425262728
Mon Feb 10 2020 10:20:00 GMT-0700 (Mountain Standard Time)14151617181920212223242526272829
Mon Feb 10 2020 10:40:00 GMT-0700 (Mountain Standard Time)15161718192021222324252627282930
Mon Feb 10 2020 11:00:00 GMT-0700 (Mountain Standard Time)16171819202122232425262728293031
Mon Feb 10 2020 11:20:00 GMT-0700 (Mountain Standard Time)17181920212223242526272829303132
Mon Feb 10 2020 11:40:00 GMT-0700 (Mountain Standard Time)18192021222324252627282930313233
Charlie
Mon Feb 10 2020 06:00:00 GMT-0700 (Mountain Standard Time)12345678910111213141516
Mon Feb 10 2020 06:20:00 GMT-0700 (Mountain Standard Time)234567891011121314151617
Mon Feb 10 2020 06:40:00 GMT-0700 (Mountain Standard Time)3456789101112131415161718
Mon Feb 10 2020 07:00:00 GMT-0700 (Mountain Standard Time)45678910111213141516171819
Mon Feb 10 2020 07:20:00 GMT-0700 (Mountain Standard Time)567891011121314151617181920
Mon Feb 10 2020 07:40:00 GMT-0700 (Mountain Standard Time)6789101112131415161718192021
Mon Feb 10 2020 08:00:00 GMT-0700 (Mountain Standard Time)78910111213141516171819202122
Mon Feb 10 2020 08:20:00 GMT-0700 (Mountain Standard Time)891011121314151617181920212223
Mon Feb 10 2020 08:40:00 GMT-0700 (Mountain Standard Time)9101112131415161718192021222324
Mon Feb 10 2020 09:00:00 GMT-0700 (Mountain Standard Time)10111213141516171819202122232425
Mon Feb 10 2020 09:20:00 GMT-0700 (Mountain Standard Time)11121314151617181920212223242526
Mon Feb 10 2020 09:40:00 GMT-0700 (Mountain Standard Time)12131415161718192021222324252627
Mon Feb 10 2020 10:00:00 GMT-0700 (Mountain Standard Time)13141516171819202122232425262728
Mon Feb 10 2020 10:20:00 GMT-0700 (Mountain Standard Time)14151617181920212223242526272829
Mon Feb 10 2020 10:40:00 GMT-0700 (Mountain Standard Time)15161718192021222324252627282930
Mon Feb 10 2020 11:00:00 GMT-0700 (Mountain Standard Time)16171819202122232425262728293031
Mon Feb 10 2020 11:20:00 GMT-0700 (Mountain Standard Time)17181920212223242526272829303132
Mon Feb 10 2020 11:40:00 GMT-0700 (Mountain Standard Time)18192021222324252627282930313233
Don
Mon Feb 10 2020 06:00:00 GMT-0700 (Mountain Standard Time)12345678910111213141516
Mon Feb 10 2020 06:20:00 GMT-0700 (Mountain Standard Time)234567891011121314151617
Mon Feb 10 2020 06:40:00 GMT-0700 (Mountain Standard Time)3456789101112131415161718
Mon Feb 10 2020 07:00:00 GMT-0700 (Mountain Standard Time)45678910111213141516171819
Mon Feb 10 2020 07:20:00 GMT-0700 (Mountain Standard Time)567891011121314151617181920
Mon Feb 10 2020 07:40:00 GMT-0700 (Mountain Standard Time)6789101112131415161718192021
Mon Feb 10 2020 08:00:00 GMT-0700 (Mountain Standard Time)78910111213141516171819202122
Mon Feb 10 2020 08:20:00 GMT-0700 (Mountain Standard Time)891011121314151617181920212223
Mon Feb 10 2020 08:40:00 GMT-0700 (Mountain Standard Time)9101112131415161718192021222324
Mon Feb 10 2020 09:00:00 GMT-0700 (Mountain Standard Time)10111213141516171819202122232425
Mon Feb 10 2020 09:20:00 GMT-0700 (Mountain Standard Time)11121314151617181920212223242526
Mon Feb 10 2020 09:40:00 GMT-0700 (Mountain Standard Time)12131415161718192021222324252627
Mon Feb 10 2020 10:00:00 GMT-0700 (Mountain Standard Time)13141516171819202122232425262728
Mon Feb 10 2020 10:20:00 GMT-0700 (Mountain Standard Time)14151617181920212223242526272829
Mon Feb 10 2020 10:40:00 GMT-0700 (Mountain Standard Time)15161718192021222324252627282930
Mon Feb 10 2020 11:00:00 GMT-0700 (Mountain Standard Time)16171819202122232425262728293031
Mon Feb 10 2020 11:20:00 GMT-0700 (Mountain Standard Time)17181920212223242526272829303132
Mon Feb 10 2020 11:40:00 GMT-0700 (Mountain Standard Time)18192021222324252627282930313233
Earl
Mon Feb 10 2020 06:00:00 GMT-0700 (Mountain Standard Time)12345678910111213141516
Mon Feb 10 2020 06:20:00 GMT-0700 (Mountain Standard Time)234567891011121314151617
Mon Feb 10 2020 06:40:00 GMT-0700 (Mountain Standard Time)3456789101112131415161718
Mon Feb 10 2020 07:00:00 GMT-0700 (Mountain Standard Time)45678910111213141516171819
Mon Feb 10 2020 07:20:00 GMT-0700 (Mountain Standard Time)567891011121314151617181920
Mon Feb 10 2020 07:40:00 GMT-0700 (Mountain Standard Time)6789101112131415161718192021
Mon Feb 10 2020 08:00:00 GMT-0700 (Mountain Standard Time)78910111213141516171819202122
Mon Feb 10 2020 08:20:00 GMT-0700 (Mountain Standard Time)891011121314151617181920212223
Mon Feb 10 2020 08:40:00 GMT-0700 (Mountain Standard Time)9101112131415161718192021222324
Mon Feb 10 2020 09:00:00 GMT-0700 (Mountain Standard Time)10111213141516171819202122232425
Mon Feb 10 2020 09:20:00 GMT-0700 (Mountain Standard Time)11121314151617181920212223242526
Mon Feb 10 2020 09:40:00 GMT-0700 (Mountain Standard Time)12131415161718192021222324252627
Mon Feb 10 2020 10:00:00 GMT-0700 (Mountain Standard Time)13141516171819202122232425262728
Mon Feb 10 2020 10:20:00 GMT-0700 (Mountain Standard Time)14151617181920212223242526272829
Mon Feb 10 2020 10:40:00 GMT-0700 (Mountain Standard Time)15161718192021222324252627282930
Mon Feb 10 2020 11:00:00 GMT-0700 (Mountain Standard Time)16171819202122232425262728293031
Mon Feb 10 2020 11:20:00 GMT-0700 (Mountain Standard Time)17181920212223242526272829303132
Mon Feb 10 2020 11:40:00 GMT-0700 (Mountain Standard Time)18192021222324252627282930313233
Francis
Mon Feb 10 2020 06:00:00 GMT-0700 (Mountain Standard Time)12345678910111213141516
Mon Feb 10 2020 06:20:00 GMT-0700 (Mountain Standard Time)234567891011121314151617
Mon Feb 10 2020 06:40:00 GMT-0700 (Mountain Standard Time)3456789101112131415161718
Mon Feb 10 2020 07:00:00 GMT-0700 (Mountain Standard Time)45678910111213141516171819
Mon Feb 10 2020 07:20:00 GMT-0700 (Mountain Standard Time)567891011121314151617181920
Mon Feb 10 2020 07:40:00 GMT-0700 (Mountain Standard Time)6789101112131415161718192021
Mon Feb 10 2020 08:00:00 GMT-0700 (Mountain Standard Time)78910111213141516171819202122
Mon Feb 10 2020 08:20:00 GMT-0700 (Mountain Standard Time)891011121314151617181920212223
Mon Feb 10 2020 08:40:00 GMT-0700 (Mountain Standard Time)9101112131415161718192021222324
Mon Feb 10 2020 09:00:00 GMT-0700 (Mountain Standard Time)10111213141516171819202122232425
Mon Feb 10 2020 09:20:00 GMT-0700 (Mountain Standard Time)11121314151617181920212223242526
Mon Feb 10 2020 09:40:00 GMT-0700 (Mountain Standard Time)12131415161718192021222324252627
Mon Feb 10 2020 10:00:00 GMT-0700 (Mountain Standard Time)13141516171819202122232425262728
Mon Feb 10 2020 10:20:00 GMT-0700 (Mountain Standard Time)14151617181920212223242526272829
Mon Feb 10 2020 10:40:00 GMT-0700 (Mountain Standard Time)15161718192021222324252627282930
Mon Feb 10 2020 11:00:00 GMT-0700 (Mountain Standard Time)16171819202122232425262728293031
Mon Feb 10 2020 11:20:00 GMT-0700 (Mountain Standard Time)17181920212223242526272829303132
Mon Feb 10 2020 11:40:00 GMT-0700 (Mountain Standard Time)18192021222324252627282930313233