You can use one of the variations on purrr::walk
to silently map over the list of plots and save them with ggsave
. walk2
maps over two lists, or iwalk
maps over a list and its names. iwalk
is really simple if you give the plot list names; the way I did it here is a little awkward, but in the wild, such as when plotting from a data frame that's been split, or with a vector that already has names, it could be different.
aes_string
is used for creating aes
calls programmatically, but it's being deprecated in favor of tidy eval; one example of this is in the aes
docs, but I also was reminded of how to do it by this answer.
I first name the vector, with its own values as its names. Then I map over it and apply a function that takes a string y
and creates a symbol of it using rlang::sym
. This allows me to use tidy eval to create a bare column name with !!y_sum
.
# https://stackoverflow.com/a/50726130/5325862
Plots <- Ys %>%
setNames(., nm = .) %>%
map(function(y) {
y_sym <- rlang::sym(y)
ggplot(data = mtcars, aes(x = mpg, y = !!y_sym)) +
geom_point()
})
Example of one plot in the list, which can be accessed by name:
Plots$hp
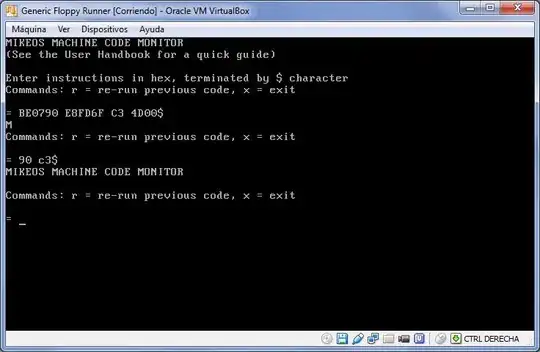
Then to save the plots, iwalk
over using the names to create filenames. You can add additional parameters to ggsave
, such as dimensions and resolution.
Plots %>%
iwalk(~ggsave(filename = sprintf("%s_vs_mpg.png", .y), plot = .x))
#> Saving 7 x 5 in image
#> Saving 7 x 5 in image
#> Saving 7 x 5 in image
#> Saving 7 x 5 in image
#> Saving 7 x 5 in image
You can do everything in one step if you need: map along to make plots and save in a single function. I went with the approach of saving the plots to a list, since that's what you did in the question, and that allows you to pull up individual plots in your document, rather than only saving them as files.