The example command line you gave creates an executable file from a source file in a single command, but it's typically done in two steps:
g++ -c -std=c++11 filesystem-testing.cpp # creates filesystem-testing.o
g++ filesystem-testing.o -lstdc++fs # creates a.out
The first step invokes the compiler, the second step the linker (g++
is the driver for both).
Note that the -lstdc++fs
flag belongs to the linker command, not the compiler command.
Eclipse's managed build system also performs the compilation in these two steps. Accordingly, you need to specify -lstdc++fs
in the linker options (e.g. Tool Settings | GCC C++ Linker | Linker flags
).
UPDATE: OK, I tried this, and I see that adding the flag to Linker flags
is not sufficient.
To understand why, let's look at the output in the Console view:
23:13:04 **** Incremental Build of configuration Debug for project test ****
Info: Internal Builder is used for build
g++ -std=c++0x -O0 -g3 -Wall -c -fmessage-length=0 -o test.o ../test.cpp
g++ -lstdc++fs -o test test.o
test.o: In function `main':
/home/nr/dev/projects/c++/test/Debug/../test.cpp:7: undefined reference to `std::experimental::filesystem::v1::current_path[abi:cxx11]()'
collect2: error: ld returned 1 exit status
23:13:05 Build Failed. 1 errors, 0 warnings. (took 880ms)
Notice it shows you the commands it's running. The linker command it's running is:
g++ -lstdc++fs -o test test.o
This is different from what I wrote above in one important way: the -lstdc++fs
options is before the input file that requires it (test.o
), rather than after. Order matters for the GCC linker.
To fix this, we need to get Eclipse to change the order of arguments to the build system. You can do this by modifying Tool Settings | GCC C++ Linker | Expert settings | Command line pattern
. This is basically a pattern for how Eclipse constructs the linker command line. Its default value is:
${COMMAND} ${FLAGS} ${OUTPUT_FLAG} ${OUTPUT_PREFIX}${OUTPUT} ${INPUTS}
Notice how ${FLAGS}
(which is where the -lstdc++fs
goes) comes before ${INPUTS}
(which is where the test.o
goes).
Let's reorder that to be:
${COMMAND} ${OUTPUT_FLAG} ${OUTPUT_PREFIX}${OUTPUT} ${INPUTS} ${FLAGS}
And try building again:
23:20:26 **** Incremental Build of configuration Debug for project test ****
Info: Internal Builder is used for build
g++ -o test test.o -lstdc++fs
23:20:26 Build Finished. 0 errors, 0 warnings. (took 272ms)
All is well now!
UPDATE 2: The following worked as that highlighted in the snapshot. It's important not to add -l
:
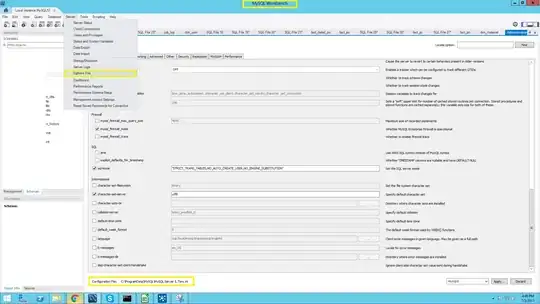