The following code shows how to ascertain the installed fonts that support displaying this group of Unicode characters (3 out of over 250 fonts installed here), and displaying them in a text area.
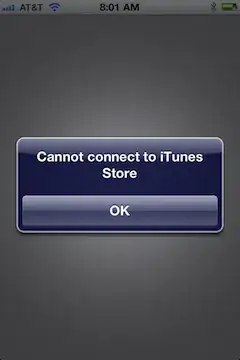
import java.awt.*;
import javax.swing.*;
import javax.swing.event.*;
import javax.swing.border.EmptyBorder;
import java.util.Vector;
public class DominoTiles {
private JComponent ui = null;
public static final int DOMINO_TILE_START = 127024;
public static final int DOMINO_TILE_END = 127123;
String dominoeTiles;
JTextArea textArea = new JTextArea(4, 10);
DominoTiles() {
initUI();
}
public void initUI() {
if (ui != null) {
return;
}
ui = new JPanel(new BorderLayout(4, 4));
ui.setBorder(new EmptyBorder(4, 4, 4, 4));
StringBuilder sb = new StringBuilder();
for (int ii = DOMINO_TILE_START; ii <= DOMINO_TILE_END; ii++) {
String s = new String(Character.toChars(ii));
sb.append(s);
}
textArea.setText(sb.toString());
textArea.setLineWrap(true);
ui.add(new JScrollPane(textArea));
String[] fontFamilies = GraphicsEnvironment.
getLocalGraphicsEnvironment().getAvailableFontFamilyNames();
final Vector<String> compatibleFonts = new Vector<>();
for (String fontFamily : fontFamilies) {
Font f = new Font(fontFamily, Font.PLAIN, 1);
if (f.canDisplayUpTo(sb.toString()) < 0) {
compatibleFonts.add(fontFamily);
}
}
final JList list = new JList(compatibleFonts);
ui.add(new JScrollPane(list), BorderLayout.LINE_START);
ListSelectionListener listSelectionListener = new ListSelectionListener() {
@Override
public void valueChanged(ListSelectionEvent e) {
if (!e.getValueIsAdjusting()) {
String fontFamily = list.getSelectedValue().toString();
Font f = new Font(fontFamily, Font.PLAIN, 60);
textArea.setFont(f);
}
}
};
list.addListSelectionListener(listSelectionListener);
list.setSelectedIndex(0);
list.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
}
public JComponent getUI() {
return ui;
}
public static void main(String[] args) {
Runnable r = new Runnable() {
@Override
public void run() {
try {
UIManager.setLookAndFeel(UIManager.getSystemLookAndFeelClassName());
} catch (Exception useDefault) {
}
DominoTiles o = new DominoTiles();
JFrame f = new JFrame(o.getClass().getSimpleName());
f.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
f.setLocationByPlatform(true);
f.setContentPane(o.getUI());
f.pack();
f.setMinimumSize(f.getSize());
f.setVisible(true);
}
};
SwingUtilities.invokeLater(r);
}
}
This example shows how to turn Unicode characters into images (plus a few more tricks of rendering them).
