Taking up John Stud's question here, because I've had the same 'problem'. In the following, I'll comment on how to read in a shapefile, hexagonize it with H3, and get a Hexagon geodataframe from it (and eventually save it as a shapefile).
Reproducible example
Let's get a shapefile for the US, e.g. here (I use the "cb_2018_us_state_500k.zip" one).
# Imports
import h3
import geopandas as gpd
import matplotlib.pyplot as plt
import pandas as pd
import shapely
from shapely.ops import unary_union
from shapely.geometry import mapping, Polygon
# Read shapefile
gdf = gpd.read_file("data/cb_2018_us_state_500k.shp")
# Get US without territories / Alaska + Hawaii
us = gdf[~gdf.NAME.isin(["Hawaii", "Alaska", "American Samoa",
"United States Virgin Islands", "Guam",
"Commonwealth of the Northern Mariana Islands",
"Puerto Rico"])]
# Plot it
fig, ax = plt.subplots(1,1)
us.plot(ax=ax)
plt.show()
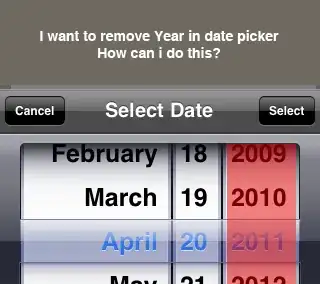
# Convert to EPSG 4326 for compatibility with H3 Hexagons
us = us.to_crs(epsg=4326)
# Get union of the shape (whole US)
union_poly = unary_union(us.geometry)
# Find the hexagons within the shape boundary using PolyFill
hex_list=[]
for n,g in enumerate(union_poly):
if (n+1) % 100 == 0:
print(str(n+1)+"/"+str(len(union_poly)))
temp = mapping(g)
temp['coordinates']=[[[j[1],j[0]] for j in i] for i in temp['coordinates']]
hex_list.extend(h3.polyfill(temp,res=5))
# Create hexagon data frame
us_hex = pd.DataFrame(hex_list,columns=["hex_id"])
# Create hexagon geometry and GeoDataFrame
us_hex['geometry'] = [Polygon(h3.h3_to_geo_boundary(x, geo_json=True)) for x in us_hex["hex_id"]]
us_hex = gpd.GeoDataFrame(us_hex)
# Plot the thing
fig, ax = plt.subplots(1,1)
us_hex.plot(ax=ax, cmap="prism")
plt.show()
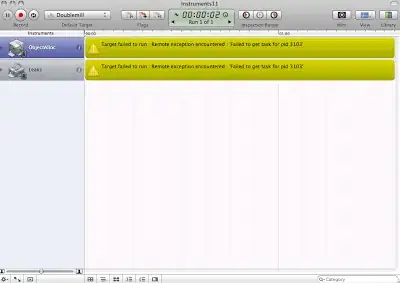
The above plot has resolution "5" (https://h3geo.org/docs/core-library/restable/), I suggest you look at other resolutions, too, like 4:
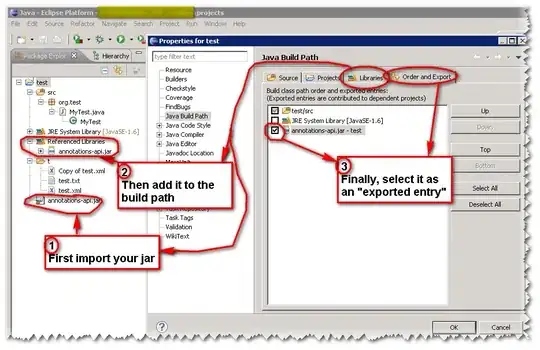
Of course, that depends on the "zoom level", i.e., whether you're looking at entire countries or just cities or so.
And, of course, to answer the original question: You can save the resulting shapefile using
us_hex.to_file("us_hex.shp")