If you want to remove communities while maintaining the other existing communities you cannot create an induced subgraph with vertices you want to keep and cluster on the subgraph because the resulting communities can very likely change.
A workable approach would be to manually subset the communities object.
Also, if you want to plot the original graph and communities and new ones and maintain the same colors everywhere you have to do a couple additional steps.
suppressPackageStartupMessages(library(igraph))
set.seed(123)
g <- erdos.renyi.game(50, 0.05)
c <- cluster_louvain(g)
l <- layout_with_fr(g)
c_keep_ids <- as.numeric(names(sizes(c)[sizes(c) >= 5]))
c_keep_v_idxs <- which(c$membership %in% c_keep_ids)
g_sub <- induced_subgraph(g, V(g)[c_keep_v_idxs])
# igraph has no direct functionality to subset community objects so hack it
c_sub <- c
c_sub$names <- c$names[c_keep_v_idxs]
c_sub$membership <- c$membership[c_keep_v_idxs]
c_sub$vcount <- length(c_sub$names)
c_sub$modularity <- modularity(g_sub, c_sub$membership, E(g_sub)$weight)
par(mfrow = c(1, 2))
plot(c, g,
layout = l,
vertex.label = NA,
vertex.size = 5
)
plot(c_sub, g_sub,
col = membership(c)[c_keep_v_idxs],
layout = l[c_keep_v_idxs, ],
mark.border = rainbow(length(communities(c)), alpha = 1)[c_keep_ids],
mark.col = rainbow(length(communities(c)), alpha = 0.3)[c_keep_ids],
vertex.label = NA,
vertex.size = 5
)
par(mfrow = c(1, 1))
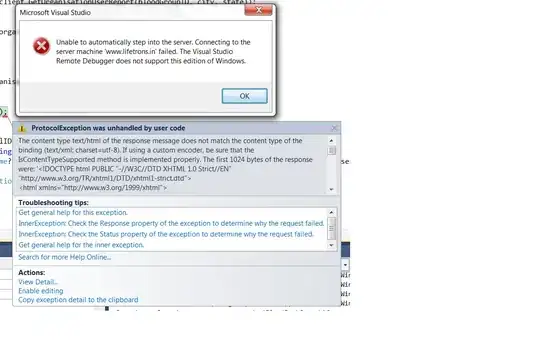