I am not really sure where are you trying to call stringByEvaluatingJavaScriptFromString
in your code, you should call it once the webView finishes loading, because you are trying to modify the UI HTML page (by changing font) if you try to run your JavaScript command even before webpage finishes loading, though your javaScript code runs, it wont be able to modify the properties of its components because they might not yet have finished rendering.
Hence,
Step 1:
Make your ViewController the navigationDelegate
of your webView
webView.navigationDelegate = self
Step 2:
Add extension to your ViewController and confirm to WKNavigationDelegate
extension ViewController : WKNavigationDelegate {
func webView(_ webView: WKWebView, didFinish navigation: WKNavigation!) {
let changeFontFamilyScript = "document.getElementsByTagName(\'body\')[0].style.fontFamily = \"Impact,Charcoal,sans-serif\";"
webView.evaluateJavaScript(changeFontFamilyScript) { (response, error) in
debugPrint("Am here")
}
}
}
Step 3:
Observe the usage of '\' to escape special characters like singleQuote (') and doubleQuotes (") in "document.getElementsByTagName(\'body\')[0].style.fontFamily = \"Impact,Charcoal,sans-serif\";"
which you are missing in your code above
Step 4:
Make sure your provide proper FontaFamilies and options to fall back if your webPage cant find the specified FontFamily (Look at 3 font names I have specified Impact,Charcoal,sans-serif)
O/P
Without NavigationDelegate (without running javaScript)
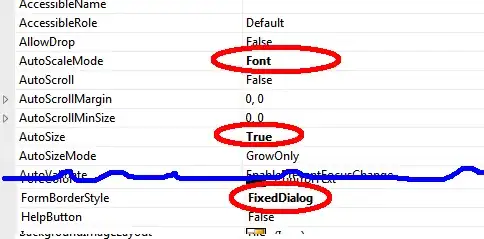
After Applying Font
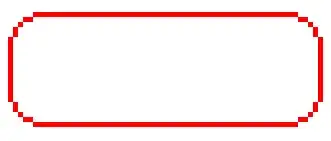
Hope this helps