The quick answer is that you can use
DataFrame.resample().mean().interpolate()
To at least do the interpolation part of your post.
Note that your post includes "out of domain" extrapolation, as you are predicting outside the domain of the input data. i.e the time series starts at 5:00 AM on 1/7, however your oversampled data starts 5 hours earlier. Interpolation is only a within domain method, but I suspect that is what you wanted.
Here's the steps for interpolating.
First, it helps if you can post a self contained example with code that either generates data for testing, or has some way to reproduce it.
Reference these two excellent posts:
Combine Date and Time columns using python pandas
How to create a Pandas DataFrame from a string
Here's how I did it:
import pandas as pd
from io import StringIO
from bokeh.plotting import figure, output_notebook, show
# copied and pasted from your post :)
data = StringIO("""
Date Time Entry Exist
2013-01-07 05:00:00 29.0 12.0
2013-01-07 10:00:00 98.0 83.0
2013-01-07 15:00:00 404.0 131.0
2013-01-07 20:00:00 2340.0 229.0
2013-01-08 05:00:00 3443.0 629.0
2013-01-08 10:00:00 6713.0 1629.0
2013-01-08 15:00:00 9547.0 2965.0
2013-01-08 20:00:00 10440.0 4589.0""")
# read in the data, converting the separate date and times to a single date time.
# see the link to do this "after the fact" if your data has separate date and time columns
df = pd.read_csv(data,
parse_dates={"date_time": ['Date', 'Time']},
delim_whitespace=True)
Now, make the data a time series, resample it, apply a function (mean in this case) and interpolate both data columns at the same time.
df_rs = df.set_index('date_time').resample('H').mean().interpolate('linear')
df_rs
Which looks like this:

Those values don't look exactly like the ones in your post, but it was not clear what sort of interpolation was being used. Linear, Cubic? Other?
So for fun, let's plot the data with bokeh. Large red dots are the orig data, while the blue dots (and connecting lines) are the interpolated data.
output_notebook()
p = figure(x_axis_type="datetime", width=800, height=500)
p.title.text = "Entry vs. Date Time (cubic interpolated to 1H)"
p.xaxis.axis_label = 'Date Time (cubic interpolated to 1H)'
p.yaxis.axis_label = 'Entry'
# orig data
p.circle(df['date_time'], df['Entry'], color='red', size=10)
# oversampled data
p.circle(df_rs.index, df_rs['Entry'])
p.line(df_rs.index, df_rs['Entry'])
show(p)
Which looks like this:
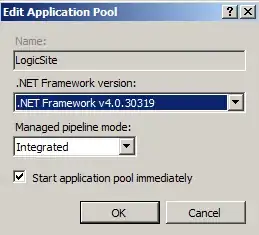
Or with cubic interpolation, you get a bit more smoothing:
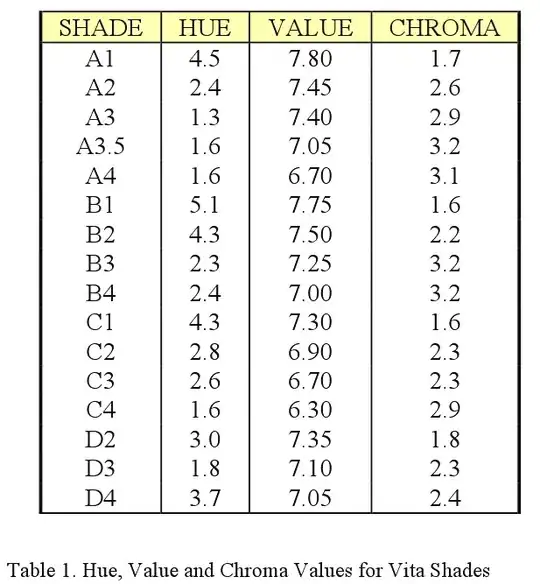
FULL CODE
import pandas as pd
from io import StringIO
from bokeh.plotting import figure, output_notebook, show
output_notebook()
# copied and pasted from your post :)
data = StringIO("""
Date Time ENTRIES EXITS
2013-01-07 05:00:00 29.0 12.0
2013-01-07 10:00:00 98.0 83.0
2013-01-07 15:00:00 404.0 131.0
2013-01-07 20:00:00 2340.0 229.0
2013-01-08 05:00:00 3443.0 629.0
2013-01-08 10:00:00 6713.0 1629.0
2013-01-08 15:00:00 9547.0 2965.0
2013-01-08 20:00:00 10440.0 4589.0""")
# read in the data, converting the separate date and times to a single date time.
# see the link to do this "after the fact" if your data as separate date and time columns
original_data = pd.read_csv(data,
parse_dates={"DATETIME": ['Date', 'Time']},
delim_whitespace=True)
# make it a time series, resample to a higher freq, apply mean, interpolate and round
inter_data = original_data.set_index(['DATETIME']).resample('H').mean().interpolate('linear').round(1)
# No need to drop the index to select a slice. You can slice on the index
# I see you are starting at 1/1 (jan 1st), yet your data starts at 1/7 (Jan 7th?)
inter_data[inter_data.index >= '2013-01-01 00:00:00'].head(20)
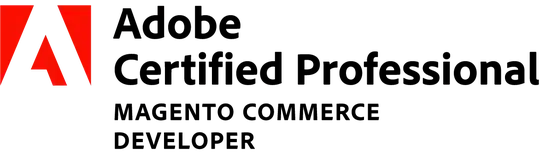