I faced same problem with textView
i used custom indent & it working fine-
@IBOutlet var bulletTextView: UITextView!
override func viewDidLoad() {
let bullet1 = "This is a small string,This is a small string,This is a small string,This is a small string,This is a small string,This is a small string,This is a small string"
let bullet2 = "This is more of medium string with a few more words etc."
let bullet3 = "Well this is certainly a longer string, with many more words than either of the previuos two strings"
strings = [bullet1, bullet2, bullet3]
let fullAttributedString = NSMutableAttributedString()
for string: String in strings {
let attributesDictionary:[NSAttributedStringKey:Any] = [NSAttributedStringKey.font : bulletTextView.font,NSAttributedStringKey.foregroundColor : UIColor.red]
let bulletPoint: String = "\u{2022}"
//let formattedString: String = "\(bulletPoint) \(string)\n"
let attributedString = NSMutableAttributedString(string: bulletPoint, attributes: attributesDictionary)
attributedString.append(NSAttributedString(string: " \(string) \n"))
let indent:CGFloat = 15
let paragraphStyle = createParagraphAttribute(tabStopLocation: indent, defaultTabInterval: indent, firstLineHeadIndent: indent - 10, headIndent: indent)
attributedString.addAttributes([NSAttributedStringKey.paragraphStyle: paragraphStyle], range: NSMakeRange(0, attributedString.length))
fullAttributedString.append(attributedString)
}
bulletTextView.attributedText = fullAttributedString
}
func createParagraphAttribute(tabStopLocation:CGFloat, defaultTabInterval:CGFloat, firstLineHeadIndent:CGFloat, headIndent:CGFloat) -> NSParagraphStyle {
let paragraphStyle: NSMutableParagraphStyle = NSParagraphStyle.default.mutableCopy() as! NSMutableParagraphStyle
let options:[NSTextTab.OptionKey:Any] = [:]
paragraphStyle.tabStops = [NSTextTab(textAlignment: .left, location: tabStopLocation, options: options)]
paragraphStyle.defaultTabInterval = defaultTabInterval
paragraphStyle.firstLineHeadIndent = firstLineHeadIndent
paragraphStyle.headIndent = headIndent
return paragraphStyle
}
Output:-
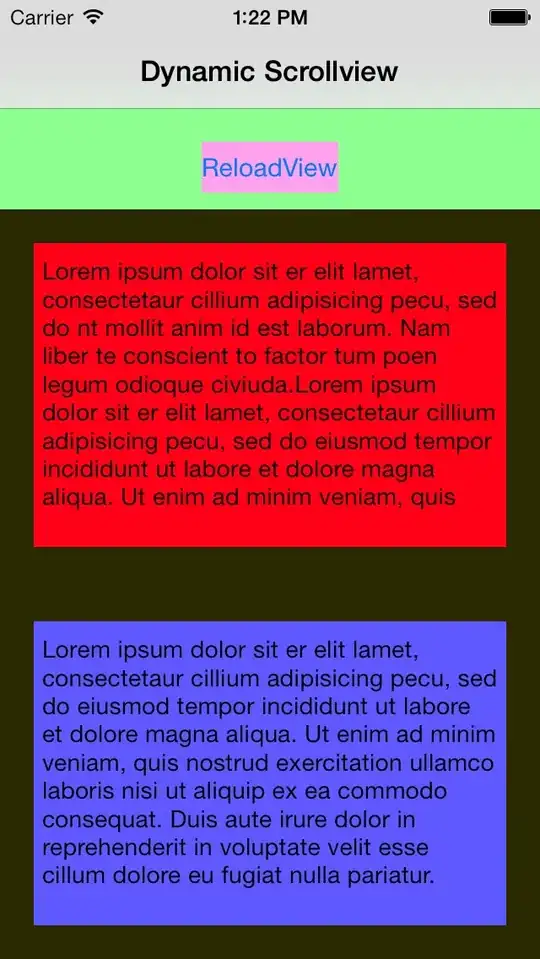