You can use tableHTML
for that:
library(tableHTML)
The mtcars
dataset is use throughout this answer:
Create a tableHTML
object using the tableHTML()
function. Then apply conditional css, if the column (in this case the rownames, i.e. index 0
) contains
a specific word. The css that is applied is simply highlighting the background using yellow:
mtcars %>%
tableHTML() %>%
add_css_conditional_column(columns = 0,
conditional = "contains",
value = "Toyota",
css = list(c("background-color"),
c("yellow")))
The result is:
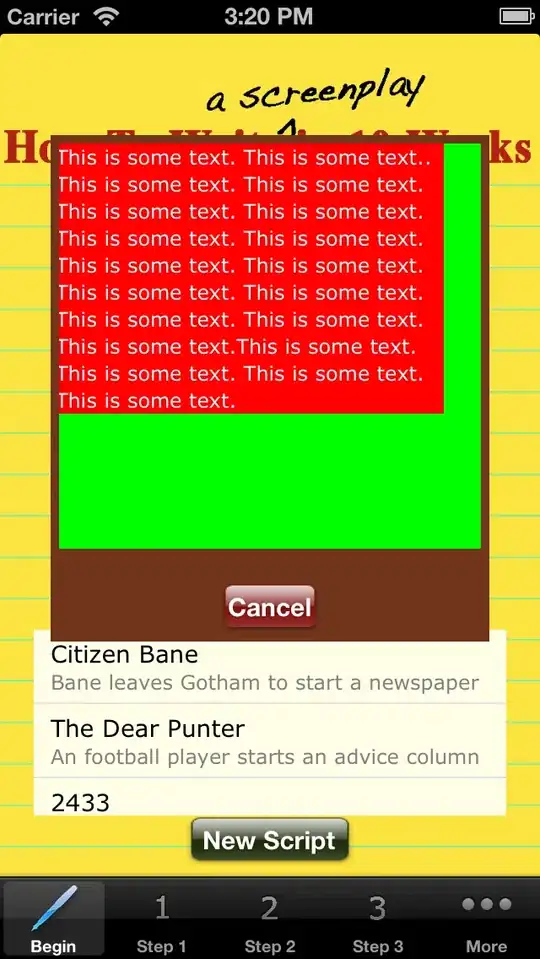
In case of many words that should be matched, you can create a vector of words:
words <- c("Merc", "Fiat", "Honda")
Create the basic tableHTML
object:
tableHTML <- mtcars %>%
tableHTML()
And apply the css word for word using a loop:
for (word in words) {
tableHTML <- tableHTML %>%
add_css_conditional_column(columns = 0,
conditional = "contains",
value = word,
css = list(c("background-color"),
c("yellow")))
}
The result is:
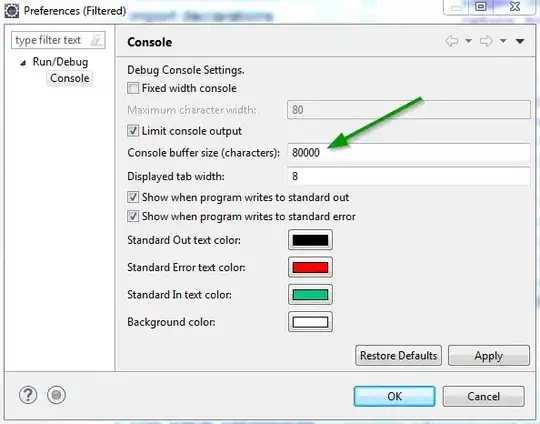
If you only want to highlight a certain substring, you could modify the data and include a span
around the substring and apply css there.
library(magrittr) # for the %<>% pipe
rownames(mtcars) %<>%
stringr::str_replace_all(c('Merc' = '<span style="background-color:yellow">Merc</span>',
'Fiat' = '<span style="background-color:yellow">Fiat</span>',
'Honda' = '<span style="background-color:yellow">Honda</span>'))
mtcars %>%
tableHTML()
The result is:
