Your NvApplicationContext
needs to be told about any form instance that you want the context class to manage; it does not happen automatically.
I can think of multiple ways to structure this:
- Allow your constructor to take multiple
Form
instances, using params
- Add a RegisterForm method to
NvApplicationContext
and call whenever you create a form that needs to be monitored
- Add a CreateForm method to
NvApplicationContext
and use it to create a form that needs to be monitored
- In the class library, create a base form class that registers itself on creation, and have your
Form1
and Form2
inherit from that base class
Allow constructor to take multiple Form
s
In the class library:
public class NvApplicationContext : ApplicationContext {
public NvApplicationContext(params Form[] forms) {
// register event handler on each form here
}
}
and used like this in the end projects:
var nvca = new NvApplicationContext(new Form1(), new Form2(), new Form3());
Application.Run(nvca);
Using a RegisterForm
method
Define a static instance of NvApplicationContext
:
public class NvApplicationContext : ApplicationContext {
public static readonly NvApplicationContext Current = new NvApplicationContext();
public void RegisterForm(Form frm) {
// register event handler on form here
}
}
and call RegisterForm
once you've created the form. This could be before the call to Application.Run
:
NvApplicationContext.Current.RegisterForm(new Form1());
NvApplicationContext.Current.RegisterForm(new Form2());
NvApplicationContext.Current.RegisterForm(new Form3());
Application.Run(NvApplicationContext.Current);
or anywhere in the application, because NvApplicationContext.Current
is a static
field and globally available.
Using a CreateForm
method
Another option is to create any form that needs to be managed, via NvApplicationContext
, and it will take care of registering the form. This assumes a static
instance of the context, as in the previous section:
public class NvApplicationContext : ApplicationContext {
public static readonly NvApplicationContext Current = new NvApplicationContext();
public T CreateForm<T>() where T : Form, new() {
var ret = new T();
// register event handlers here
return ret;
}
}
and then call before Application.Run
NvApplicationContext.Current.CreateForm<Form1>();
NvApplicationContext.Current.CreateForm<Form2>();
NvApplicationContext.Current.CreateForm<Form3>();
Application.Run(NvApplicationContext.Current);
Using a base form
You could write a base form class like this (either in your class library, or in the end project), calling RegisterForm
with a static
instance from before:
public class BaseForm : Form {
public BaseForm() {
NvApplicationContext.Current.RegisterForm(this);
}
}
and create forms that inherit from the base class either with the UI:
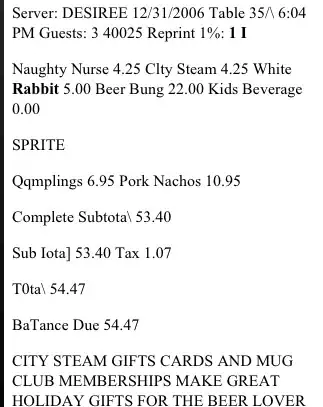
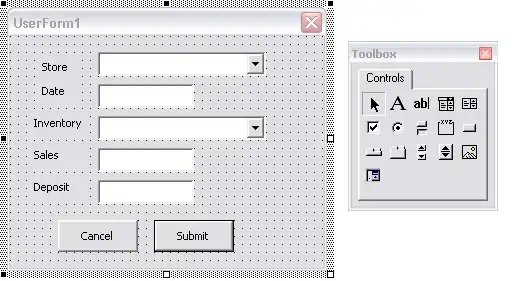
Or, you could do this from the code-behind of the form. Modify the base class from Form
to BaseForm
:
public partial class Form1 : BaseForm {
public Form1() {
InitializeComponent();
}
}
Once you've set up the inheritance, whenever you create a new instance of the form it will automatically be registered with NvApplicationContext.Current
.
new Form1();
new Form2();
new Form3();
Application.Run(NvApplicationContext.Current);