Let me clarify few things here as it can be confusing.
When using user flows, the easiest way to define custom attribute is using Azure portal. You have to open user flows blade in the Azure AD B2C, and select User attributes:
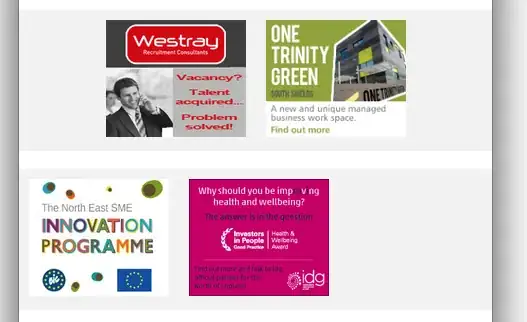
Once you add the custom attribute, it will be visible on the list. From this point you can decide whether it should be returned in the token to the application or not. To include custom attribute value in the token, you have to
open Application claims tab and select your custom attribute added before:
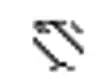
Now when it comes to attribute value. You mentioned that you would like to fulfill this value as admin using Graph API. Here is the fragment of my web app to manage users with .NET Microsoft Graph Client:
public async Task UpdateUserAsync(UserAccount userAccount, CancellationToken cancellationToken)
{
try
{
string myCustomAttributeForUserAttributeName = _adB2cCustomAttributeHelper.GetCompleteAttributeName("myCustomAttributeForUser");
IDictionary<string, object> extensionsInstance = new Dictionary<string, object>();
if (userAccount.myCustomAttributeForUser != null)
{
extensionsInstance.Add(myCustomAttributeForUserAttributeName, userAccount.myCustomAttributeForUser);
}
var user = new User
{
AdditionalData = extensionsInstance
};
await _graphServiceClient.Users[existingUser.Id]
.Request()
.UpdateAsync(user, cancellationToken);
}
catch (ServiceException ex)
{
if (ex.StatusCode == System.Net.HttpStatusCode.TooManyRequests)
{
var retryAfter = ex.ResponseHeaders.RetryAfter.Delta.Value;
...
}
else
{
_logger.LogError...
}
}
}
Important
In the source code above, as you can see I am using _adB2cCustomAttributeHelper insatnce. This is because when you use Microsoft Graph API, you have to provide full name of the custom attribute which also consists of extensions application's ID registered in the Azure AD B2C:

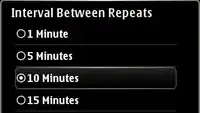
You can read more about it here. Custom attribute is stored in this format (wthout '-'):
extension_<<extension_app_client_id>_AttributeName
This is AdB2cCustomAttributeHelper class definition:
public class AdB2cCustomAttributeHelper
{
internal readonly string _b2cExtensionAppClientId;
public AdB2cCustomAttributeHelper(string b2cExtensionAppClientId)
{
_b2cExtensionAppClientId = b2cExtensionAppClientId.Replace("-", "");
}
internal string GetCompleteAttributeName(string attributeName)
{
if (string.IsNullOrWhiteSpace(attributeName))
{
throw new System.ArgumentException("Parameter cannot be null", nameof(attributeName));
}
return $"extension_{_b2cExtensionAppClientId}_{attributeName}";
}
}
I register above instance as singleton and I pass extensions application ID to it as a parameter:
services.AddSingleton(implementationFactory =>
{
var options = implementationFactory.GetRequiredService<IOptions<MsGraphServiceConfiguration>>();
return new AdB2cCustomAttributeHelper(options.Value.ADB2CExtensionAppId);
});
Under this link you can also see another example with adding extension attribute to the user's account using Microsoft Graph API.
When you sign in, you will see the extension attribute in the token:
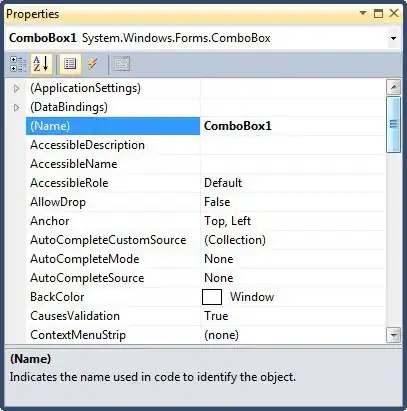