My approach to this is the following:
1). Get the device height. Also check this answer.
DisplayMetrics displayMetrics = new DisplayMetrics();
getWindowManager().getDefaultDisplay().getMetrics(displayMetrics);
int height = displayMetrics.heightPixels;
2). Calculate the ratio.
You have set a top margin of 130dp
I assume. Using pixplicity I managed to convert it to px
.
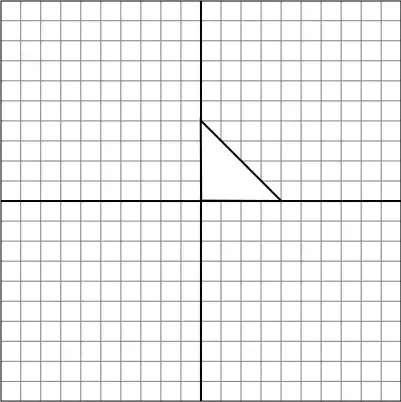
Your phone has a resolution height x weight in pixels. Let's define height as phoneHeight
.
Here it becomes a little tricky for me too. As you can see 130dp has different pixel values for ldpi, mdpi, etc. I personally don't know which one you should use, you might try the mdpi one. (I'll try to research more and come back with an answer).
LE:
You can use this method to convert dp to pixels according to your phone. (See this answer).
public static float convertDpToPixel(float dp, Context context){
Resources resources = context.getResources();
DisplayMetrics metrics = resources.getDisplayMetrics();
float px = dp * ((float)metrics.densityDpi / DisplayMetrics.DENSITY_DEFAULT);
return px;
}
Use the returned px into the formula below. (let's call the returned px - convertedPx
.
3). The formula for the top margin's value.
If we use the value of 130dp for mdpi from the table we get 130px. So the formula would be:
margin_top = (convertedPx / phoneHeight) * height
with phoneHeight
the height of the phone you are currently testing on and height
the height of the device using displayMetrics
.
NOTICE: convertedPx
will be a constant. You only have to run this method (convertDpToPixel
) on your phone to find out how much 130dp mean in pixels.