Here is a rather simple approach.
Background:
Dominant colors like red, green, blue and yellow can be segmented pretty easily when analyzed in LAB color space. LAB space has 3 channels, out of which only 2 are color channels while 1 is a brightness channel:
- L-channel: represents the brightness
- A-channel: represents the amount of red/green color
- B-channel: represents the amount of blue/yellow color
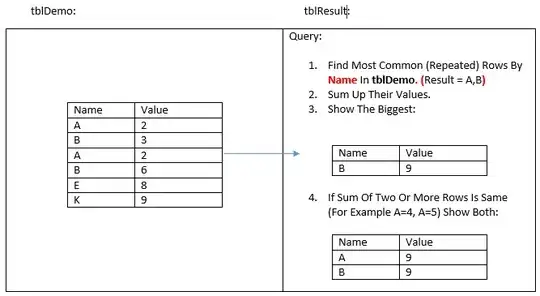
Observing the plot above:
- a-axis clearly shows red/green color on its extremes
- b-axis shows blue/yellow color on its extremes
Applying a suitable threshold in the corresponding channels makes it easy for us to segment these colors.
Approach:
We will use the above info as a base to approach the problem at hand:
1. Perform basic masking:
->
Convert image to LAB space
->
Threshold a-channel to isolate green background
->
Masking original image with binary mask
img = cv2.imread('green_background.jpg')
lab = cv2.cvtColor(img, cv2.COLOR_BGR2LAB)
a_channel = lab[:,:,1]
th = cv2.threshold(a_channel,127,255,cv2.THRESH_BINARY+cv2.THRESH_OTSU)[1]
masked = cv2.bitwise_and(img, img, mask = th) # contains dark background
m1 = masked.copy()
m1[th==0]=(255,255,255) # contains white background
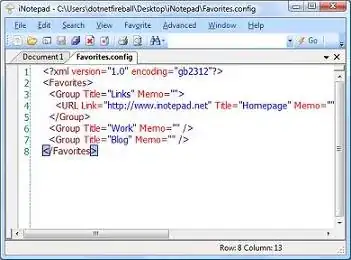
2. Remove green shade along the border:
->
Convert masked image LAB space
->
Normalize the a-channel mlab[:,:,1]
to use the entire intensity range between [0-255]
->
Inverse binary threshold to select area with green borders
mlab = cv2.cvtColor(masked, cv2.COLOR_BGR2LAB)
dst = cv2.normalize(mlab[:,:,1], dst=None, alpha=0, beta=255,norm_type=cv2.NORM_MINMAX, dtype=cv2.CV_8U)
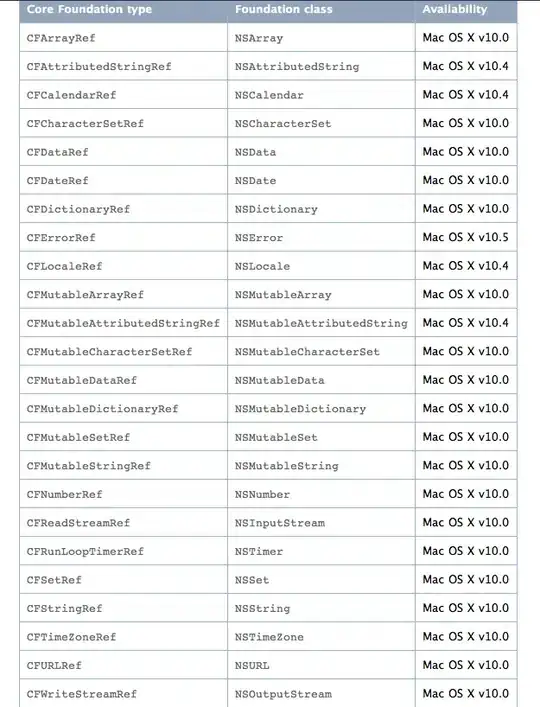
Above image is the a-channel with dark pixels around the border.
threshold_value = 100
dst_th = cv2.threshold(dst, threshold_value, 255, cv2.THRESH_BINARY_INV)[1]
Above is the threshold image to segment green shaded regions.
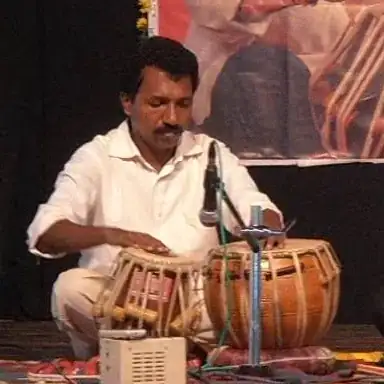
In the a-channel, having green color represents the lower end of the range [0-255], while red color represents the higher end. We will set intensity value in the a-channel of the selected pixels to 127:
mlab2 = mlab.copy()
mlab[:,:,1][dst_th == 255] = 127
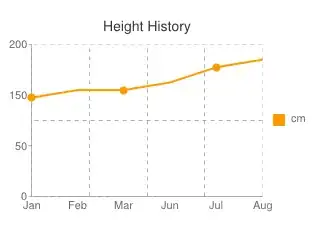
Now in the above image, we do not see the dark borders around the person.
Convert the image to BGR and the pixels that were dark (0) in the threshold image are set to white (255, 255, 255) in the color image
img2 = cv2.cvtColor(mlab, cv2.COLOR_LAB2BGR)
img2[th==0]=(255,255,255)
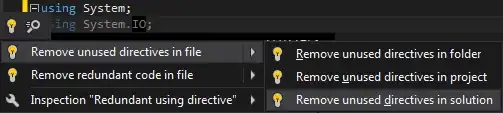
The resulting image looks like nothing much has changed, so here is a zoomed in comparison:
Before Step 2:
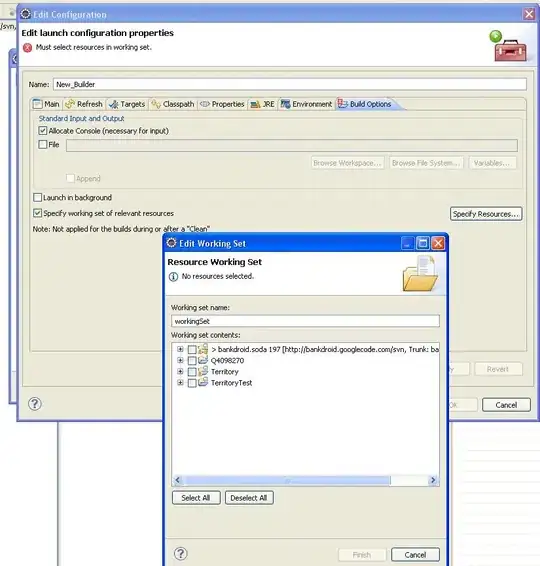
After Step 2:
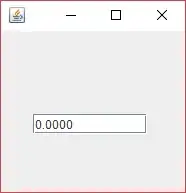
Conclusion:
The noisy green outline around the border has improved to a certain extent. You can try varying threshold_value
and experiment.
The green shade present within the person's face still remains. Sophisticated methods will be needed to get rid of those. Hope this approach helps.