If your project is in WindowsFormApplicaiton
then
If your control is button
like in screen shot
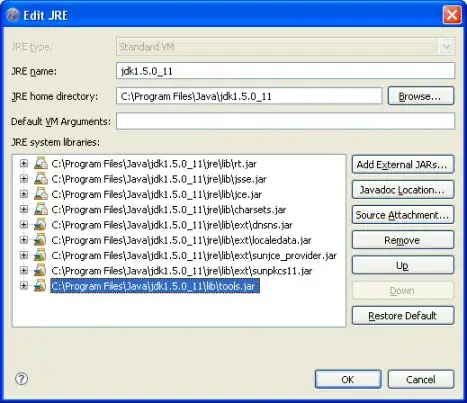
Then you can access its location wrt to its primary screen also and form screen also
Below is the code
private void button1_Click(object sender, EventArgs e)
{
//This code gives you the location of button1 wrt your primary working screen
Point location = this.PointToScreen(button1.Location);
int x1 = location.X;
int y1 = location.Y;
MessageBox.Show($"X: {x1}, Y: {y1}");
//This code gives you the location of button1 wrt your forms upper-left corner
Point relativeLoc = new Point(location.X - this.Location.X, location.Y - this.Location.Y);
int x2 = relativeLoc.X;
int y2 = relativeLoc.Y;
MessageBox.Show($"X: {x2}, Y: {y2}");
//This code gives you the location of button1 wrt your forms client area
Point relativeLoc1 = new Point(button1.Location.X, button1.Location.Y);
int x3 = relativeLoc1.X;
int y3 = relativeLoc1.Y;
MessageBox.Show($"X: {x3}, Y: {y3}");
}
In above code I used this
, you can use any forms
object instead as your need
Edit:
If you don't know where your control will be clicked then you have to register one event for all of your controls inside forms like
In below code i used MouseHover
event but you can used any event as your need
First of all register MouseHover
event for all of your controls inside Form1_Load
like
private void Form1_Load(object sender, EventArgs e)
{
foreach (Control c in this.Controls)
c.MouseHover += myMouseHoverEvent;
}
Then your custom MouseHover
event is
private void myMouseHoverEvent(object sender, EventArgs e)
{
Control control = sender as Control;
int x = control.Location.X;
int y = control.Location.Y;
MessageBox.Show($"X: {x}, Y: {y}");
}
Try once may it help you