Ok, I adapted this from an MDN example to do a very simple and most likely inaccurate download speed test. It runs 100% on the browser and since everything is local for now speeds are too fast, so in Chrome you can enable throttling to get a more realistic experience.
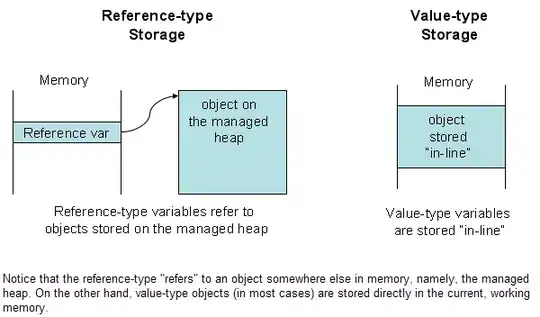
In the server I have a text.txt file that weights about 3 MB. On the same server and path I have the following code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Document</title>
</head>
<body>
<div>
Progress: <span class="progress">0%</span>
</div>
<div>
File size: <span class="size">...</span>
</div>
<div>
Total time: <span class="time">...</span>
</div>
<div>
Speed: <span class="speed">...</span>
</div>
<script>
const start = Date.now();
const url = 'text.txt';
const xhr = new XMLHttpRequest();
let end;
let size;
let time;
xhr.addEventListener("progress", updateProgress);
xhr.addEventListener("load", transferComplete);
xhr.addEventListener("error", transferFailed);
xhr.addEventListener("abort", transferCanceled);
xhr.open('GET', url);
xhr.send();
function updateProgress(oEvent) {
if (oEvent.lengthComputable) {
if (!size) {
size = oEvent.total;
document.querySelector('.size').innerHTML = `${Number.parseFloat(size / 1024 / 1024).toFixed(2)} MB`;
}
var percentComplete = oEvent.loaded / oEvent.total * 100;
document.querySelector('.progress').innerHTML = `${parseInt(percentComplete, 10)}%`;
}
}
function transferComplete(evt) {
console.log('The transfer is complete.');
end = Date.now();
time = (end - start) / 1000;
document.querySelector('.time').innerHTML = `${parseInt(time, 10)} seconds`;
document.querySelector('.speed').innerHTML = `${Number.parseFloat(size / time / 1024).toFixed(2)} kB/s`;
}
function transferFailed(evt) {
console.log('An error occurred while transferring the file.');
}
function transferCanceled(evt) {
console.log('The transfer has been canceled by the user.');
}
</script>
</body>
</html>