The problem could be parameter sniffing, maybe not. I'll skip that topic since @KumarHarsh already covered it. The most important question here is: What data type is FactOrderLines.DD_OrderDate? This is important for performance reasons as well as correctness.
First for performance. If DD_OrderDate is a DATE
datatype and your variables or parameters are DATETIME then the optimizer has to jump through extra hoops to utilize your index or will be forced to do a scan instead of a seek. Note the following sample data:
USE tempdb;
GO
IF OBJECT_ID('#FactOrderLines') IS NOT NULL DROP TABLE #FactOrderLines;
GO
CREATE TABLE #FactOrderLines(someId INT IDENTITY, DD_OrderDate DATETIME NOT NULL);
CREATE CLUSTERED INDEX nc_factOrderLines ON #FactOrderLines(DD_OrderDate);
INSERT #FactOrderLines(DD_OrderDate)
SELECT TOP (10000) DATEADD(DAY,CHECKSUM(NEWID())%100, getdate())
FROM sys.all_columns;
GO
Now let's compare the execution plans for the following queries:
-- AS DATE
DECLARE @StartDate DATE = '2018-08-01',
@EndDate DATE = '2018-08-20';
SELECT *
FROM #FactOrderLines
WHERE DD_OrderDate >= @StartDate
AND DD_OrderDate <= @EndDate
OPTION (RECOMPILE)
GO
-- AS DATETIME
DECLARE @StartDate DATETIME = '2018-08-01',
@EndDate DATETIME = '2018-08-31';
SELECT *
FROM #FactOrderLines
WHERE DD_OrderDate >= @StartDate
AND DD_OrderDate <= @EndDate
OPTION (RECOMPILE);
Execution plans:
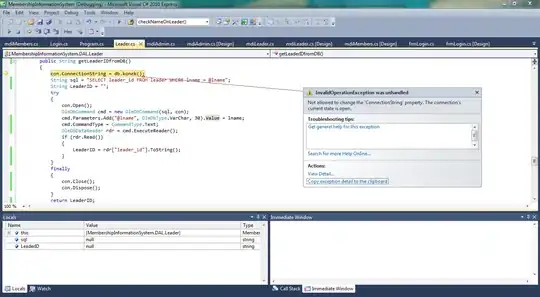
For this reason - you want to make sure that you're using the same datatype for your variables/parameters as for the column they are working against.
Now about correctness; note this query:
DECLARE @StartDate DATE = '2018-08-01',
@EndDate DATE = '2018-08-20';
SELECT
[getdate as datetime] = GETDATE(),
[@enddate as datetime] = CAST(@EndDate AS DATETIME),
[getdate as date] = CAST(GETDATE() AS DATE),
[datetime equality] = IIF(GETDATE() > @EndDate,'yep','nope'),
[date equality] = IIF(CAST(GETDATE() AS DATE) > @EndDate,'yep','nope');
Results:
getdate as datetime @enddate as datetime getdate as date datetime equality date equality
----------------------- ----------------------- --------------- ----------------- -------------
2018-08-20 13:52:46.247 2018-08-20 00:00:00.000 2018-08-20 yep nope
Values of the date format translate into datetime as 0 hour, 0 second...