As of now, you cannot rotate 3D plots generated by MatPlotlib in Colab. Plotly is a better alternative.
However, you can use the view_init
method to change the view. It has two parameters (elev
for elevation and azim
for azimuth angles) to set to rotate the axes. You will get a different viewpoint.
By default, the view_init
is set as azim=-60, elev=30
. The angles are specified in degrees.
In your code, change the values of elev
and azim
to something else before using the scatter method. An example that uses ax.view_init(elev=30, azim=-120)
is shown below:
import matplotlib.pyplot as plt
import numpy as np
from mpl_toolkits.mplot3d import axes3d
fig = plt.figure()
ax = fig.add_subplot(111, projection = '3d')
x1 = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
y1 = np.random.randint(10, size=10)
z1 = np.random.randint(10, size=10)
x2 = [-1, -2, -3, -4, -5, -6, -7, -8, -9, -10]
y2 = np.random.randint(-10, 0, size=10)
z2 = np.random.randint(10, size=10)
ax.view_init(elev=30, azim=-120)
ax.scatter(x1, y1, z1, c='b', marker='o', label='blue')
ax.scatter(x2, y2, z2, c='g', marker='D', label='green')
ax.set_xlabel('x axis')
ax.set_ylabel('y axis')
ax.set_zlabel('z axis')
plt.title("3D Scatter Plot Example")
plt.legend()
plt.tight_layout()
plt.show()
This produces the following plot:
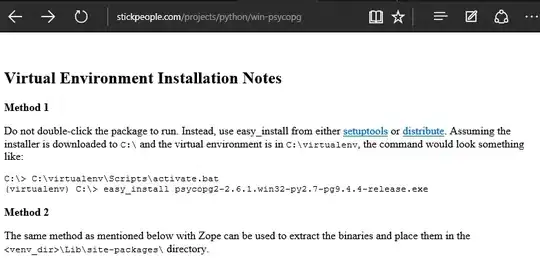
Note:
ax.view_init(elev=0, azim=60)
: A zero elevation makes the plot as if we are looking horizontally.
ax.view_init(elev=-30, azim=60)
: A negative elevation inverts vertically.
This figure explains the meaning of azimuth and elevation better.
