A plus 1 to the guys suggesting cte's. You're probably going to find that their suggestions are going to be the best option. The code I use for this is usually wrapped in a user defined function, but I've stripped it out for an example here:
DECLARE @str NVARCHAR(MAX) = 'This,Is,A,Sentence'
, @Delimiter NCHAR(1) = ',';
WITH cte AS
(SELECT 1 AS ID
, CASE WHEN CHARINDEX(@Delimiter, @str, 1) = 0 THEN @str
ELSE LEFT(@str, CHARINDEX(@Delimiter, @str, 1) - 1)
END Words
, CASE WHEN CHARINDEX(@Delimiter, @str, 1) = 0 THEN ''
ELSE STUFF(@str, 1, CHARINDEX(@Delimiter, @str, 1), '')
END Remainder
UNION ALL
SELECT cte.ID + 1
, CASE WHEN CHARINDEX(@Delimiter, cte.Remainder, 1) = 0 THEN cte.Remainder
ELSE LEFT(cte.Remainder, CHARINDEX(@Delimiter, cte.Remainder, 1) - 1)
END
, CASE WHEN CHARINDEX(@Delimiter, cte.Remainder, 1) = 0 THEN ''
ELSE STUFF(cte.Remainder, 1, CHARINDEX(@Delimiter, cte.Remainder, 1), '')
END Remainder
FROM cte
WHERE Remainder <> '')
SELECT cte.ID [Index]
, cte.Words
FROM cte;
Result set:
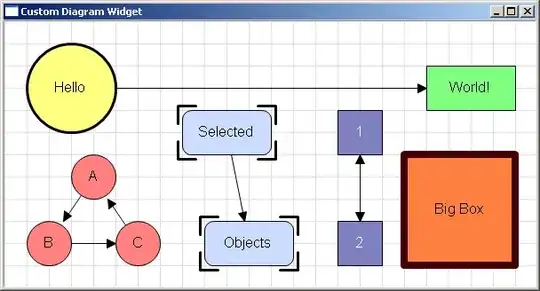
You can of course strip out the id/index column if you're not going to need it
Incidentally, if you compare this to the built in Split function in the latest version of SQL Server, this is actually far more efficient. Running both together cte version 16% of load, built in function 84%. That's a big difference.
