As I suggested in the comments, I created a div
, initially hidden, that plays the role of a popup modal that will be showed when an a
tag that has a data-target="modal"
is clicked. I made some changes to your HTML
code that I will cover them in the answer.
The process:
When you click a link that has data-target="modal"
(a link that has an href
attribute of a video, here I added a data-target="modal"
to each one just to differentiate these links from the other links that may be present in the page) the modal div
that i gave it id="modal"
will be showed and filled with the appropriete video. So, each time a link is clicked its href
attribute will be placed in the src
attribute of the iframe
tag that will host the video.
The final result:
Ps: I'll provide a runnable snippet, but, it may not work as intended due to some restrictions made by Stack Overflow. For that, I made a working DEMO on CodePen that you can check and play with the code, if you want.
var modal = document.getElementById('modal'), closeBtn = modal.querySelector('.close'), ytVideo = modal.querySelector('.content .yt-video'), title = modal.querySelector('.content .title'), anchors = document.querySelectorAll('a[data-target="modal"]'), l = anchors.length;
for (var i = 0; i < l; i++) {
anchors[i].addEventListener("click", function(e) {
e.preventDefault();
title.textContent = this.dataset.videoTitle || 'No title';
ytVideo.src = this.href;
modal.classList.toggle('is-visible');
modal.focus();
});
}
modal.addEventListener("keydown", function(e) {
if (e.keyCode == 27) {
title.textContent = '';
ytVideo.src = '';
this.classList.toggle('is-visible');
}
});
closeBtn.addEventListener("click", function(e) {
e.preventDefault();
title.textContent = '';
ytVideo.src = '';
modal.classList.toggle('is-visible');
});
#modal {
display: none;
position: fixed;
width: 100vw;
height: 100vh;
max-height: 100vh;
top: 0;
left: 0;
background: rgba(24, 24, 24, .6);
z-index: 999;
}
#modal .content {
width: 55%;
height: 65vh;
margin: auto; /* allows horyzontal and vertical alignment as .content is in flex container */
}
#modal .content .yt-video {
display: block;
width: 100%;
height: calc(100% - 45px);
}
#modal .content .title {
box-sizing: border-box;
height: 45px;
line-height: 23px;
padding: 12px 4px;
margin: 0;
background: #007bff;
max-width: 100%;
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
}
#modal .close {
position: absolute;
top: 0;
right: 0;
width: 35px;
height: 35px;
line-height: 35px;
text-align: center;
border: 0;
font-weight: bold;
font-size: 24px;
color: #fff;
background: #666;
cursor: pointer;
transition: background .4s;
}
#modal .close:hover, #modal .close:active {
background: #ef3658;
}
#modal.is-visible {
display: flex;
}
<ul id="toolsButtons" class="ahrefs__tools__nav">
<li>
<div class="tools-icon">
<a href="https://www.youtube.com/embed/A1nRiiWYgZw" target="_blank" data-target="modal" data-video-title="Traditional vs GATE"><img src="Traditional_vs_GATE_s.png"></a>
<use xlink:href="#keyword-research-icon"></use>
<p class="tools-icon__text">Traditional vs GATE</p>
</li>
<li>
<div class="tools-icon">
<a href="https://www.youtube.com/embed/wLHfGQlLqtE" target="_blank" data-target="modal" data-video-title="GATE Passcode"><img src="Guess_GATE_Password.png"></a>
<use xlink:href="#competitive-analysis-icon"></use>
<p class="tools-icon__text">GATE Passcode</p>
</div>
</li>
<li>
<div class="tools-icon">
<a href="https://www.youtube.com/embed/5tAGemIvUeI" target="_blank" data-target="modal" data-video-title="How GATE Works"><img src="GATE_Demo.png"></a>
<use xlink:href="#keyword-research-icon"></use>
<p class="tools-icon__text">How GATE Works</p>
</div>
</li>
</ul>
<!-- the modal div that will open when an anchor link is clicked to show the related video in an iframe. -->
<div id="modal" tabindex="-1">
<button type="button" data-dismiss="modal" class="close" title="close">×</button>
<div class="content">
<h4 class="title"></h4>
<iframe class="yt-video" src="https://www.youtube.com/embed/A1nRiiWYgZw" frameborder="0" allow="autoplay; encrypted-media" allowfullscreen></iframe>
</div>
</div>
Changes that must be done in the HTML
code:
- Each
a
tag that holds an href
attribute for a video that you want it to be showed in the modal must have a data-target
attribute equals to "modal".
- To display a title above the video when the modal
div
is showed, add a data-video-title
attribute that equals to some text on these a
tags. If the data-video-title
is not present on a link, the title that appears above the video will be "No title".
- As an illustration to the last 2 points, here's an example of a link that will open the modal when clicked:
<a href="https://www.youtube.com/embed/A1nRiiWYgZw" target="_blank" data-target="modal" data-video-title="Traditional vs GATE"><img src="Traditional_vs_GATE_s.png"></a>
- All the videos links (in other words the
href
attribute of the a
tags) MUST be an embed
link (https://www.youtube.com/embed/A1nRiiWYgZw
as an example). So, you have to use the link in the src
of the iframe
under the embed
section under share
section on YouTube. Here are some images to demonstrate that:
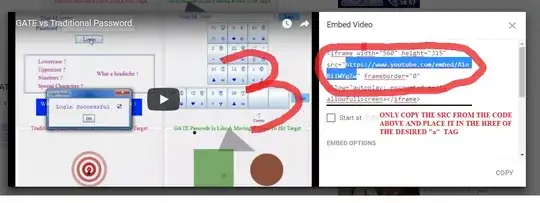
Why using embed
instead of the regular watch
link ?
That to prevent Cross-Domain-Policy restrictions. In simple words, by default servers don't allow other servers/sites to fetch their files asynchronously.
Notes on the modal div
- For simplicity, I didn't done any animations as opening/closing effects, and the modal isn't responsive.
- You can press
ESC
(Escape) button to close the modal.
- A button with a "cross" sign will be placed on the top-right most of the browser window, to close the modal on click.
- The markup of the modal
div
must be reserved to work as intended.
Why i didn't use data-href
instead of href
?
I sticked with the href
on the links to garantee that the video will be accessible even if JavaScript
is disabled, if so, it will be redirected to the video.
Hope my answer pushed you further. I'm here for any further explanations.