I got it all to work. Thanks for the big help. Much apprieciated!
I will show it all if any can use any off it :D
Ended up with this:
using (SqlConnection Con = new SqlConnection(Connectionstring.selectedBrugerString))
{
Con.Open();
SqlCommand cmd = new SqlCommand("SELECT USERNAME FROM PERSONAL", Con);
using (SqlDataReader reader = cmd.ExecuteReader())
{
while (reader.Read())
{
fromComboBox.Items.Add(reader[0]);
toComboBox.Items.Add(reader[0]);
}
}
var countUsers = fromComboBox.Items.Count;
movePostMessage.Text = countUsers + " brugere er fundet";
}
}
And on the button click i've made this:
using (SqlConnection Con = new SqlConnection(Connectionstring.selectedBrugerString))
{
Con.Open();
string SQL1 = "UPDATE DEBSALDO SET PERSONALID = (SELECT PERSONALID FROM PERSONAL WHERE USERNAME = '" + toComboBox.Text + "') WHERE PERSONALID IN (SELECT PERSONALID FROM PERSONAL WHERE USERNAME = '" + fromComboBox.Text + "')";
string SQL2 = "UPDATE SERVHEAD SET PERSONALID = (SELECT PERSONALID FROM PERSONAL WHERE USERNAME = '" + toComboBox.Text + "') WHERE PERSONALID IN (SELECT PERSONALID FROM PERSONAL WHERE USERNAME = '" + fromComboBox.Text + "')";
string SQL3 = "UPDATE SERVICEB SET PERSONALID = (SELECT PERSONALID FROM PERSONAL WHERE USERNAME = '" + toComboBox.Text + "') WHERE PERSONALID IN (SELECT PERSONALID FROM PERSONAL WHERE USERNAME = '" + fromComboBox.Text + "')";
string SQL4 = "UPDATE PERSONAL SET ACOUNTCYID = NULL WHERE PERSONALID IN (SELECT PERSONALID FROM PERSONAL WHERE USERNAME = '" + fromComboBox.Text + "')";
SqlCommand cmd1 = new SqlCommand(SQL1, Con);
SqlCommand cmd2 = new SqlCommand(SQL2, Con);
SqlCommand cmd3 = new SqlCommand(SQL3, Con);
SqlCommand cmd4 = new SqlCommand(SQL4, Con);
Int32 rowsAffectedOnUpdate1 = cmd1.ExecuteNonQuery();
Int32 rowsAffectedOnUpdate2 = cmd2.ExecuteNonQuery();
Int32 rowsAffectedOnUpdate3 = cmd3.ExecuteNonQuery();
Int32 rowsAffectedOnUpdate4 = cmd4.ExecuteNonQuery();
movePostMessage.Text = rowsAffectedOnUpdate1 + " regninger flyttet fra tidligere brugers debitor saldo \r\n" + rowsAffectedOnUpdate2 + " regninger flyttet med tidligere bruger som ejer \r\n" + rowsAffectedOnUpdate3 + " ydelser flyttet med tidligere bruger som ejer \r\n" + rowsAffectedOnUpdate4 + " kontoplan nulstillet";
}
And the GUI to it:
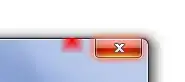