That's a common rounding error, since binary can't represent some decimal numbers. Have a look here.
Here is an online converter from a decimal to a floating-point representation. As you can see there is an error (6.103515625E-8
) due to the conversion:
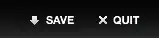
As to the difference between when you print a float directly, or when you print an object that has a property that is a float, it comes down to the description
property of objects that implement the CustomStringConvertible
protocol. When the print
function wants to print to the console, it invokes _print_unlocked, which is an internal function that has this block:
if case let printableObject as CustomStringConvertible = value {
printableObject.description.write(to: &target)
return
}
So you could change your code to this in order to have the expected output:
struct FloatContainer : CustomStringConvertible {
var description: String {
get {
return f.description
}
}
var f: Float
}