Using a System.IO.MemoryStream
adds strings to the clipboard without the extra bytes at the beginning and at the end of string values.
For example, the following string represents a fragment of a Microsoft Excel Spreadsheet:
class Program
{
[STAThread]
static void Main(string[] args)
{
string content = @"<?xml version=""1.0""?>
<?mso-application progid=""Excel.Sheet""?>
<Workbook xmlns=""urn:schemas-microsoft-com:office:spreadsheet""
xmlns:o=""urn:schemas-microsoft-com:office:office""
xmlns:x=""urn:schemas-microsoft-com:office:excel""
xmlns:ss=""urn:schemas-microsoft-com:office:spreadsheet""
xmlns:html=""http://www.w3.org/TR/REC-html40"">
<Styles>
<Style ss:ID=""Default"" ss:Name=""Normal"">
<Alignment ss:Vertical=""Bottom""/>
<Borders/>
<Font ss:FontName=""Calibri"" x:Family=""Swiss"" ss:Size=""11"" ss:Color=""#000000""/>
<Interior/>
<NumberFormat/>
<Protection/>
</Style>
<Style ss:ID=""s18"" ss:Name=""Currency"">
<NumberFormat
ss:Format=""_("$"* #,##0.00_);_("$"* \(#,##0.00\);_("$"* "-"??_);_(@_)""/>
</Style>
<Style ss:ID=""s64"">
<Font ss:FontName=""Calibri"" x:Family=""Swiss"" ss:Size=""11"" ss:Color=""#000000""
ss:Bold=""1""/>
</Style>
<Style ss:ID=""s65"" ss:Parent=""s18"">
<Font ss:FontName=""Calibri"" x:Family=""Swiss"" ss:Size=""11"" ss:Color=""#000000""/>
</Style>
</Styles>
<Worksheet ss:Name=""Sheet1"">
<Table ss:ExpandedColumnCount=""2"" ss:ExpandedRowCount=""2""
ss:DefaultRowHeight=""15"">
<Row>
<Cell><Data ss:Type=""String"">Month</Data></Cell>
<Cell><Data ss:Type=""String"">Year</Data></Cell>
</Row>
<Row>
<Cell ss:StyleID=""s64""><Data ss:Type=""String"">August</Data></Cell>
<Cell ss:StyleID=""s65""><Data ss:Type=""Number"">999.99</Data></Cell>
</Row>
</Table>
</Worksheet>
</Workbook>";
Passing it to the Clipboard
class as a string:
Clipboard.SetData("XML Spreadsheet", content);
Creates an incompatible format with Excel, and Excel shows the following error when trying to paste that content:
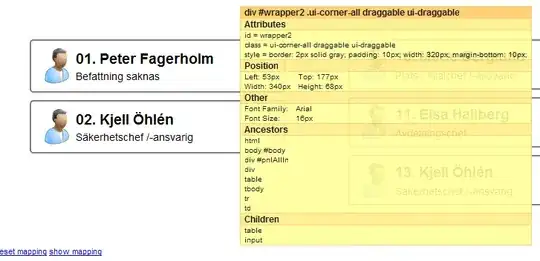
However, serializing it into bytes and then passing it as a MemoryStream:
Clipboard.SetData("XML Spreadsheet", new MemoryStream(Encoding.UTF8.GetBytes(content)));
Creates a clipboard content compatible with Excel:
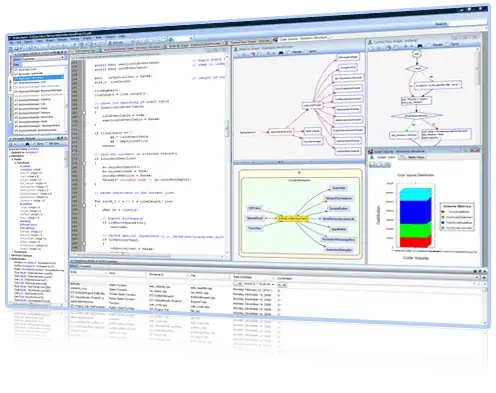