In my case I did not have the VPN connection ready to reach the external network. I was not aware that the "Network Settings" under Linux switch the VPN connection to off
after every computer restart.
And then I got this similar error:
$docker-compose up
Recreating MYCONTAINER ... done
Attaching to MYCONTAINER
MYDOCKERCOMPOSESERVICE_1 | 2022-01-14 10:56:30,045 [INFO]: Connecting to DB ...
MYDOCKERCOMPOSESERVICE_1 | Traceback (most recent call last):
MYDOCKERCOMPOSESERVICE_1 | File "/usr/lib/python3.9/runpy.py", line 197, in _run_module_as_main
MYDOCKERCOMPOSESERVICE_1 | return _run_code(code, main_globals, None,
MYDOCKERCOMPOSESERVICE_1 | File "/usr/lib/python3.9/runpy.py", line 87, in _run_code
MYDOCKERCOMPOSESERVICE_1 | exec(code, run_globals)
MYDOCKERCOMPOSESERVICE_1 | File "/MYDOCKERCOMPOSESERVICE/main.py", line 265, in <module>
MYDOCKERCOMPOSESERVICE_1 | main()
MYDOCKERCOMPOSESERVICE_1 | File "/MYDOCKERCOMPOSESERVICE/main.py", line 244, in main
MYDOCKERCOMPOSESERVICE_1 | MYSERVER_conn = create_connection(MYSERVER_CONNECTION)
MYDOCKERCOMPOSESERVICE_1 | File "/MYDOCKERCOMPOSESERVICE/main.py", line 65, in create_connection
MYDOCKERCOMPOSESERVICE_1 | conn = MySQLdb.connect(cursorclass=cursors.DictCursor, charset="utf8",
MYDOCKERCOMPOSESERVICE_1 | File "/usr/local/lib/python3.9/dist-packages/MySQLdb/__init__.py", line 123, in Connect
MYDOCKERCOMPOSESERVICE_1 | return Connection(*args, **kwargs)
MYDOCKERCOMPOSESERVICE_1 | File "/usr/local/lib/python3.9/dist-packages/MySQLdb/connections.py", line 185, in __init__
MYDOCKERCOMPOSESERVICE_1 | super().__init__(*args, **kwargs2)
MYDOCKERCOMPOSESERVICE_1 | MySQLdb._exceptions.OperationalError: (2005, "Unknown MySQL server host 'MYSERVER' (-3)")
MYDOCKERCOMPOSESERVICE_1 | (2005, "Unknown MySQL server host 'MYSERVER' (-3)")
MYCONTAINER exited with code 1
Here -3, in the question -2, I guess these are just positions in the arguments of the connection object. In my case, a connection with the db was impossible, I tested it in the bash of the container again, using mysql on the command line.
Solution: Just switch VPN on again, then the connection can be created:
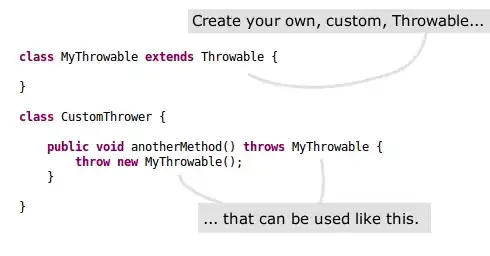
If the above does not help, you might also check whether your port 3306 is already in use by another container (docker ps -a
). If so, try ports: -"3307:3306" or another port as well. This is just a step that I also made before it ran through, though I do not think that it is related to the error in question. I still cannot say for sure whether this might not have solved something as well.