Could you please explain what you mean using non-symmetric data using this example.
Do you want negative z to be at the top side?
And why does rotating the camera not produce the result you want to see?
You can add the code from the macro editor (explained below).
import numpy as np
from mayavi import mlab
x, y, z = np.ogrid[-5:5:64j, -5:5:64j, -5:5:64j] #Generate XYZ
data = np.arange(x.shape[0])
x = x.ravel()
y = y.ravel()
z = z.ravel()
# Recorded script from Mayavi2
from numpy import array
try:
engine = mayavi.engine
except (AttributeError, NameError):
from mayavi.api import Engine
engine = Engine()
engine.start()
if len(engine.scenes) == 0:
engine.new_scene()
# -------------------------------------------
scene = engine.scenes[0]
scene.scene.camera.position = [20.68813263960946, 20.334388554161922, 20.518300376103046]
scene.scene.camera.focal_point = [0.24373197555541992, 0.24373197555541992, 0.25]
scene.scene.camera.view_angle = 30.0
scene.scene.camera.view_up = [-0.41179533881878827, -0.4046701524210215, 0.81649658092772626]
scene.scene.camera.clipping_range = [15.729834995160559, 58.864284541884331]
scene.scene.camera.compute_view_plane_normal()
scene.scene.render()
mlab.points3d(x, y, z, data) #Produce volumetric plot
mlab.axes(xlabel='X', ylabel='Y', zlabel='Z') #Display axis
mlab.orientation_axes()
mlab.show()
If you really can set the view you want manually I would just do that.
To get the correct coordinates to pass to mlab.view()
read them from the interactive plot while rotating the scene:
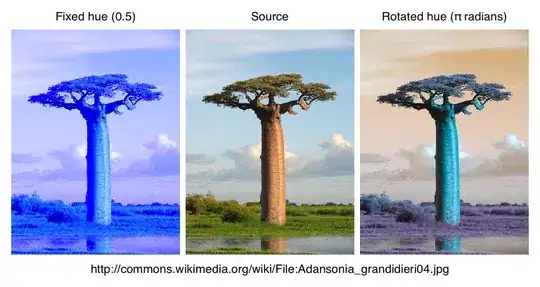