will take over the RAM
Technically, that's not possible.
RAM refers to physical memory and python.exe cannot access physical RAM. An executable in Windows only gets access to virtual memory (MSDN). How much of that is actually in RAM is decided by Windows.
For 32 bit Python, that should not be much of a problem, since it will only have access to a maximum of 4 GB. But with 64 bit of course, it will start using the page file and write to disk, which makes things slow. This will even affect other programs. For details, see memory limits (MSDN)
and freeze everything
Also, hardly possible, since Python cannot have exclusive access to the CPU. A mechanism called preemption will ensure that Windows can assign the CPU to something else.
The code you posted neither uses multithreading nor multiprocessing, so it will use only 1 CPU. If you have a single core machine, that may be at 100%, of course. On my machine, it's just 25% and everything else continues running smoothly:
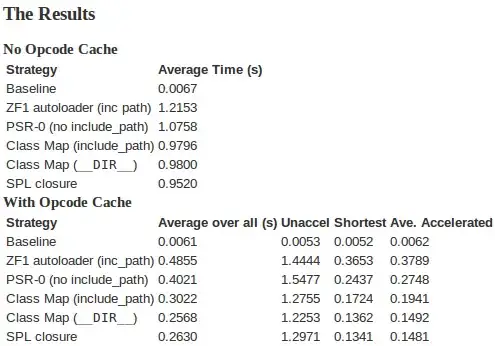
Isn't there a mechanism to limit nested raises, trys and excepts
I don't think so.
or just prevent so much memory from being allocated?
Yes, there's a mechanism called a "Job (MSDN)" in Windows which can do that. You would need to run Python.exe in a job which has a memory quota. There's a Superuser question which suggests tools for that.
As an alternative, you could of course implement a memory limit condition for the fibunacci sequence to stop, like this:
import os
import psutil
process = psutil.Process(os.getpid())
ram = psutil.virtual_memory().total
ram_limit = 70 / 100 * ram
[...]
if process.memory_info().private > ram_limit:
assert False
I used that if
for debugging reasons, so I could put a breakpoint onto the assertion to make the following screenshot.
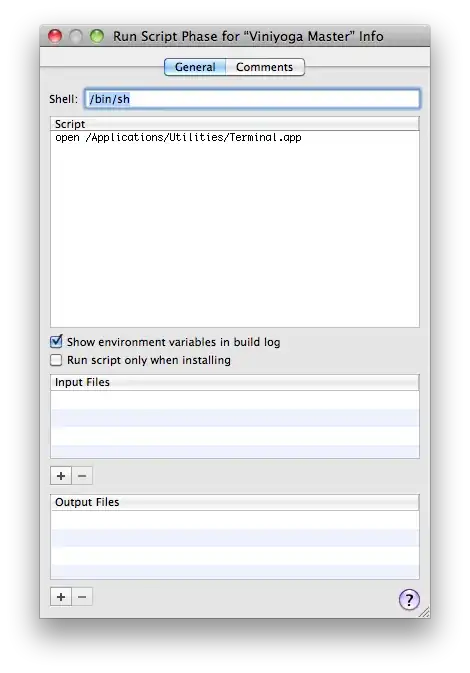
As you can see, the private bytes (memory used by the process) is ~23.5 GB and the working set (memory that fits into the computer's physical RAM) is also ~23.5 GB. No performance issues so far.