You can use trigger to avoid insertion of data into Manager table if an employee's designation is not manager.
You may want to execute this trigger on both Insertion and Updation of the records in Managers table.
CREATE OR REPLACE TRIGGER manager_validation
BEFORE INSERT OR UPDATE
ON MANAGERS_DETAILS
FOR EACH ROW
DECLARE
desig VARCHAR2(10);
BEGIN
SELECT designation
INTO desig
FROM employee
WHERE id = :new.id;
IF (desig != 'MANAGER') THEN
raise_application_error( -20001, 'Only Employees who are Managers can be inserted into table.');
END IF;
END;
Below is my working example:
-- Create Employee Table
CREATE TABLE employee_ihm
(
id NUMBER PRIMARY KEY,
name VARCHAR2(1),
designation VARCHAR2(10)
);
-- Create Managers table
CREATE TABLE managers
(
id NUMBER REFERENCES employee_ihm(id) ON DELETE CASCADE,
name VARCHAR2(1),
salary NUMBER
);
-- Create trigger on insert and update on Managers table
CREATE OR REPLACE TRIGGER manager_validation BEFORE
INSERT OR UPDATE
ON managers
FOR EACH ROW
DECLARE
desig VARCHAR2(10);
BEGIN
SELECT designation INTO desig FROM employee_ihm WHERE id = :new.id;
IF (desig != 'MANAGER') THEN
raise_application_error( -20001, 'Only Employees who are Managers can be inserted into table.');
END IF;
END;
INSERT INTO employee_ihm VALUES
(1, 'A', 'MANAGER');
INSERT INTO employee_ihm VALUES
(2, 'B', 'EMP');
INSERT INTO employee_ihm VALUES
(3, 'C', 'MANAGER');
INSERT INTO employee_ihm VALUES
(4, 'D', 'TEAMLEAD');
INSERT INTO employee_ihm VALUES
(5, 'E', 'TEAMMEM');
INSERT INTO employee_ihm VALUES
(6, 'F', 'MANAGER');
-- Employee id 1 will be inserted successfully
INSERT INTO managers VALUES
(1, 'A', 200);
-- Employee id 2 will fail to get inserted into Managers
INSERT INTO managers VALUES
(2, 'B', 300);
Let me know if this solution works.
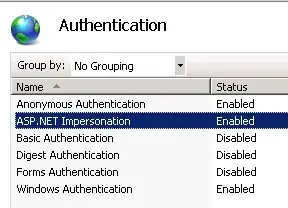
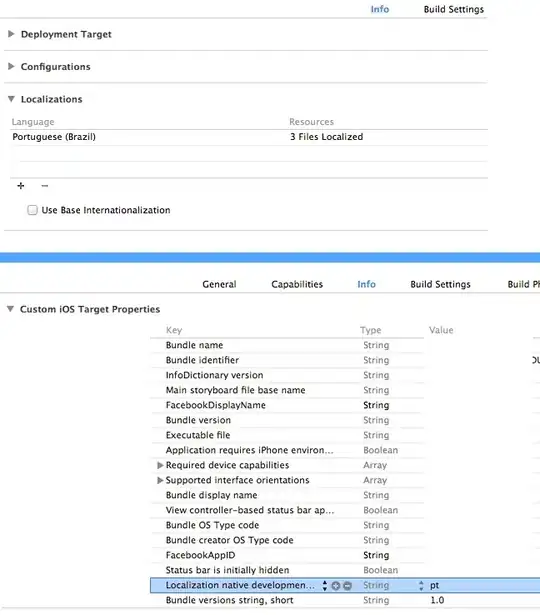