First I'd like to say: my first association with your request was matplotlib's broken_barh
function. But up to now I could not figure out how to plot timedeltas, as this would be necessary there. Your plot can be done with plot
too, so I have some code with an if False: (attempt with plt.broken_barh) else (plt.plot-version)
structure. See yourself.
I'll try to update the literally broken part, as soon as I have an idea how to plot timedeltas in matplotlib...
Here's the code I hope that can help you:
import matplotlib.pyplot as plt
import numpy as np
import pandas as pd
from io import StringIO
def brk_str(s): # just for nicer labeling with such long hashes
return '\n'.join([s[8*i:8*(i+1)] for i in range(4)])
s = ''' hash event start end
0174FAA018E7FAE1E84469ADC34EF666 baseball 00:00:00:000 00:00:00:500
0174FAA018E7FAE1E84469ADC34EF666 baseball 00:00:01:000 00:00:01:500
0174FAA018E7FAE1E84469ADC34EF666 cat 00:00:01:500 00:00:02:500
AF4BB75F98579B8C9F95EABEC1BDD988 baseball 00:00:01:000 00:00:01:500
AF4BB75F98579B8C9F95EABEC1BDD988 cat 00:00:01:500 00:00:02:500
AF4BB75F98579B8C9F95EABEC1BDD988 cat 00:00:03:200 00:00:05:250
AF4BB75F98579B8C9F95EABEC1BDD988 cat 00:00:03:000 00:00:04:350'''
df = pd.read_table(StringIO(s), sep='\s+')
df['start'] = pd.to_datetime(df['start'], format='%H:%M:%S:%f')
df['end'] = pd.to_datetime(df['end'], format='%H:%M:%S:%f')
df['dur'] = (df['end'] - df['start']) # this is only needed in case of broken_barh would work...
e_grpd = df.groupby('event')
fig, ax = plt.subplots()
for i, (e, ev) in enumerate(e_grpd): # iterate over all events, providing a counter i, the name of every event e and its data ev
last_color = None # setting color value to None which means automatically cycle to another color
for k, (h, hv)in enumerate(ev.groupby('hash')): # iterate over all hashes, providing a counter k, every hash h and its data hv
if False: # desperately not deleting this as broken_barh would save the innermost loop and would generally fit better I think...
pass
#ax.broken_barh(ev[['start', 'dur']].T, np.array([i*np.ones(len(ev))+k/10, .1*np.ones(len(ev))]).T)
else:
for n, (a, b) in enumerate(zip(hv.start, hv.end)): # iterate over every single event per hash, providing a counter n and start and stop time a and b
p = ax.plot([a, b], k*np.ones(2)+i/10, color=last_color, lw=15, label='_' if k>0 or n>0 else '' + e)
last_color = p[0].get_c() # setting color value to the last one used to prevent color cycling
ax.set_yticks(range(len(df.groupby('hash').groups)))
ax.set_yticklabels(map(brk_str, df.groupby('hash').groups))
ax.legend(ncol=2, bbox_to_anchor=[0, 0, 1, 1.1], loc=9, edgecolor='w')
plt.tight_layout()
Result with plt.plot
:
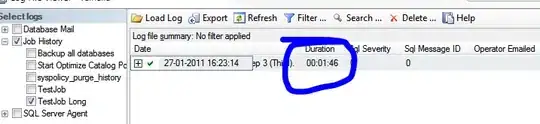