Please note that I have not studied all available libraries and just want to provide a starting point for others to solve their problems.
I will use the following localized string during all examples:
dt_str = "Thu 3 Apr 2014 13:19:52" # en_US
1. Methods of 'datetime.datetime'
This is the simplest approach, but it becomes unwieldy if you use locales other than the default locale.
Information about the syntax can be found here.
import datetime
dt = datetime.datetime.strptime(dt_str,
"%a %d %b %Y %H:%M:%S")
# datetime.datetime(2014, 4, 3, 13, 19, 52)
s = dt.strftime("%a %-d %b %Y %H:%M:%S")
# 'Thu 3 Apr 2014 13:19:52'
If you like to parse or format other locales, you have to change the global locale, which could result in undesired side effects. (I do not recommend this approach.)
import datetime
import locale
locale.setlocale(locale.LC_TIME, "de_DE.UTF-8")
dt = datetime.datetime.strptime("Do 3 Apr 2014 13:19:52",
"%a %d %b %Y %H:%M:%S")
# datetime.datetime(2014, 4, 3, 13, 19, 52)
s = dt.strftime("%a %-d %b %Y %H:%M:%S")
# 'Do 3 Apr 2014 13:19:52'
2. The python library 'arrow'
arrow allows you to pass a locale (otherwise it uses "en_US").
import arrow # installed via pip
import datetime
### localized string -> datetime
a_dt = arrow.get(dt_str,
"ddd D MMM YYYY H:mm:ss",
locale="en_US") # "en_US" is also the default, so this is just for clarification
dt = a_dt.datetime
# datetime.datetime(2014, 4, 3, 13, 19, 52, tzinfo=tzutc())
### datetime -> localized string
a_s = arrow.get(datetime.datetime(2014, 5, 17, 14, 0, 0))
s = a_s.format("ddd D MMM YYYY H:mm:ss",
locale="en_US") # "en_US" is also the default, so this is just for clarification
# 'Sat 17 May 2014 14:00:00'
This is especially useful if the desired locale differs from the default locale.
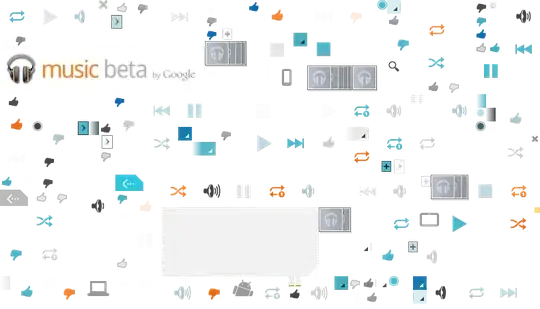
3. The python library 'babel' (mainly for formatting)
The strength of babel is the formatting (Unfortunately, it seems that it can not parse any pattern. Only the format "short" seems reliable.)
import babel.dates # 'babel' installed via pip
import datetime
dt = datetime.datetime(2014, 4, 3, 13, 19, 52)
# parsing is the problem with babel, therefore I created the datetime object directly.
s = babel.dates.format_datetime(dt,
"EEE d MMM yyyy H:mm:ss",
locale="en_US")
# 'Thu 3 Apr 2014 13:19:52'
4. The python library 'dateparser' (only parsing)
dateparser is very powerful. It is able to lookup dates in longer texts and does support non-Gregorian calendar systems, just to name a few features.
import dateparser # installed via pip
dt = dateparser.parse(dt_str,
date_formats=["%a %d %b %Y %H:%M:%S"],
languages=["en"])
# datetime.datetime(2014, 4, 3, 13, 19, 52)
5. Last but not least
The following noteworthy python libraries have great features, but unfortunately I could not use them for this specific problem (or did not know how to use them properly).