I'd recommend adding a border to your image view so you can see what's going on.
Here's what you're currently experiencing (using the imageView directly as the custom view of the bar button item):
- (void)_addImageViewToLeftButtonItemWithoutOuterView {
UIImageView *profileImageView = [[UIImageView alloc] initWithImage:[UIImage imageNamed:@"Homer.jpg"]];
[profileImageView setFrame:CGRectMake(0, 0, 40, 40)];
profileImageView.layer.cornerRadius = 20.0f;
profileImageView.layer.masksToBounds = YES;
profileImageView.clipsToBounds = YES;
profileImageView.layer.borderColor = [UIColor blackColor].CGColor;
profileImageView.layer.borderWidth = 1.0f;
UIBarButtonItem *rbi = [[UIBarButtonItem alloc] initWithCustomView:profileImageView];
self.navigationItem.leftBarButtonItem = rbi;
}
This is what it looks like without aspect fit:
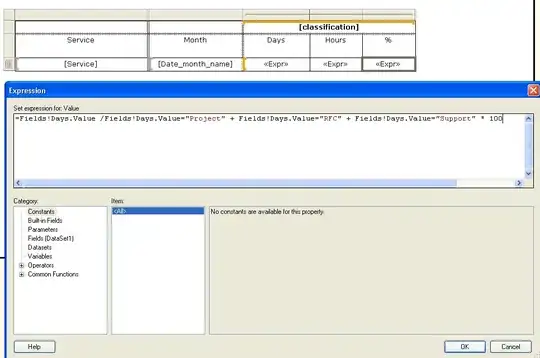
And with aspect fit set:
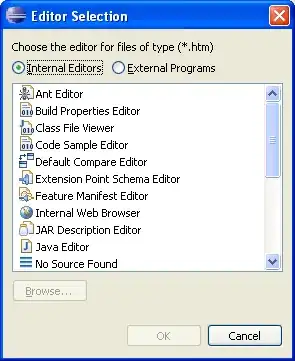
And this is what I believe you want:
- (void)_addImageViewToLeftButtonItemWithOuterView {
UIView *profileView = [[UIView alloc] initWithFrame:CGRectMake(0, 0, 40, 40)];
profileView.layer.borderColor = [[UIColor redColor] CGColor];
profileView.layer.borderWidth = 2.0f;
UIImageView *profileImageView = [[UIImageView alloc] initWithImage:[UIImage imageNamed:@"Homer.jpg"]];
profileImageView.contentMode = UIViewContentModeScaleAspectFit;
[profileImageView setFrame:CGRectMake(0, 0, 40, 40)];
profileImageView.layer.cornerRadius = 20.0f;
profileImageView.layer.masksToBounds = YES;
profileImageView.clipsToBounds = YES;
profileImageView.layer.borderColor = [UIColor blackColor].CGColor;
profileImageView.layer.borderWidth = 1.0f;
[profileView addSubview:profileImageView];
UIBarButtonItem *lbi = [[UIBarButtonItem alloc] initWithCustomView:profileView];
self.navigationItem.leftBarButtonItem = lbi;
}
Which results in this:
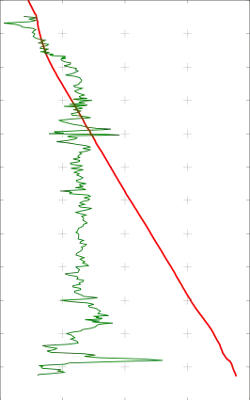
As you probably noticed, the sorta trick here is that you don't want to add the image view directly as the custom view within initWithCustomView
of the UIBarButtonItem. Instead you want to use an outer view to hold the imageView, then you can adjust the frame of your subview to move it around.
Here's one more example if you wanted the image further to the right (obviously you won't want the border for the outer image view, but I've just left it there for demonstration purposes):
- (void)_addImageViewToLeftButtonItemWithOuterView {
// adjust this to 80 width (it always starts at the origin of the bar button item
UIView *profileView = [[UIView alloc] initWithFrame:CGRectMake(0, 0, 80, 40)];
profileView.layer.borderColor = [[UIColor redColor] CGColor];
profileView.layer.borderWidth = 2.0f;
UIImageView *profileImageView = [[UIImageView alloc] initWithImage:[UIImage imageNamed:@"Homer.jpg"]];
profileImageView.contentMode = UIViewContentModeScaleAspectFit;
// and adjust this to be offset by 40 (move it to the right some)
[profileImageView setFrame:CGRectMake(40, 0, 40, 40)];
profileImageView.layer.cornerRadius = 20.0f;
profileImageView.layer.masksToBounds = YES;
profileImageView.clipsToBounds = YES;
profileImageView.layer.borderColor = [UIColor blackColor].CGColor;
profileImageView.layer.borderWidth = 1.0f;
[profileView addSubview:profileImageView];
UIBarButtonItem *lbi = [[UIBarButtonItem alloc] initWithCustomView:profileView];
self.navigationItem.leftBarButtonItem = lbi;
}
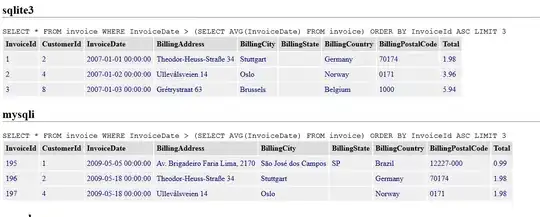