tl;dr
Use Instant
, not LocalDateTime
.
Instant // Represent a moment as seen in UTC (an offset of zero hours-minutes-seconds).
.parse( "2018-01-23T01:23:45.123456789Z" ) // Parse text in standard ISO 8601 format where the `Z` on the end means UTC.
.truncatedTo( java.time.temporal.ChronoUnit.SECONDS ) // Lop off any fractional second.
.plus( // Date-time math.
Duration.ofDays( 2 ) // Represent a span-of-time not attached to the timeline.
) // Returns another `Instant` object rather than altering the original.
.toString() // Generate text in standard ISO 8601 format.
2018-01-25T01:23:45Z
ISO 8601
parse the String in format "yyyy-MM-dd'T'HH:mm:ss'Z'" into LocalDateTime
You cannot. Or should not use LocalDateTime
for this purpose.
Your input string is in standard ISO 8601 format. The Z
on the end means UTC, and is pronounced “Zulu”. You should never ignore this character, as you seem to be intending by the use of single-quote marks around it.
Instant
Parse such a string as a Instant
, a moment in UTC.
Instant instant = Instant.parse( "2018-01-23T01:23:45.123456789Z" ) ;
LocalDateTime
The LocalDateTime
class cannot represent a moment. It purposely lacks any concept of time zone or offset-from-UTC. This class represents potential moments along a range of about 26-27 hours, the various time zones currently in use around the globe.
OffsetDateTime
To communicate with a database via JDBC, use OffsetDateTime
.
OffsetDateTime odt = instant.atOffset( ZoneOffset.UTC ) ;
ZonedDateTime
To see the moment of that Instant
through the lens of the wall-clock time used by the people of a certain region (a time zone), apply a ZoneId
to get a ZonedDateTime
object.
ZoneId z = ZoneId.of( "America/Montreal" ) ;
ZonedDateTime zdt = instant.atZone( z ) ;
Weekend
To test for the weekend, use the DayOfWeek
enum in an EnumSet
.
Set< DayOfWeek > weekend = EnumSet.of( DayOfWeek.SATURDAY , DayOfWeek.SATURDAY ) ;
For any given moment, the date varies around the globe by time zone. For example, a few minutes after midnight in Paris France is a new day, while still “yesterday” in Montréal Québec. So adjust your Instant
into the time zone appropriate to your business problem, as shown above.
Compare by extracting a DayOfWeek
enum object from your ZonedDateTime
.
DayOfWeek dow = zdt.getDayOfWeek() ;
Compare to see if it is a weekend or weekday.
ZonedDateTime target = zdt ; // Initialize.
if( weekend.contains( dow ) ) {
target = zdt.with( TemporalAdjusters.next( DayOfWeek.MONDAY ) ) ; // Move from one day to another.
}
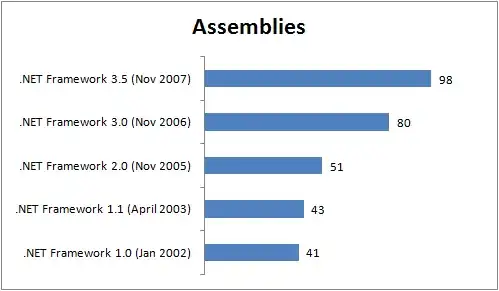
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.