Yes, We can but I am not sure it's the right way. But it works for me
First, we need to get all the qualities need for the video.
There are two ways to get this option
- Get it from the back end itself via API
- The "m3u8" you can actually download it and read its contents. All you have to do is get the value for the key "RESOLUTION"
It will be like this
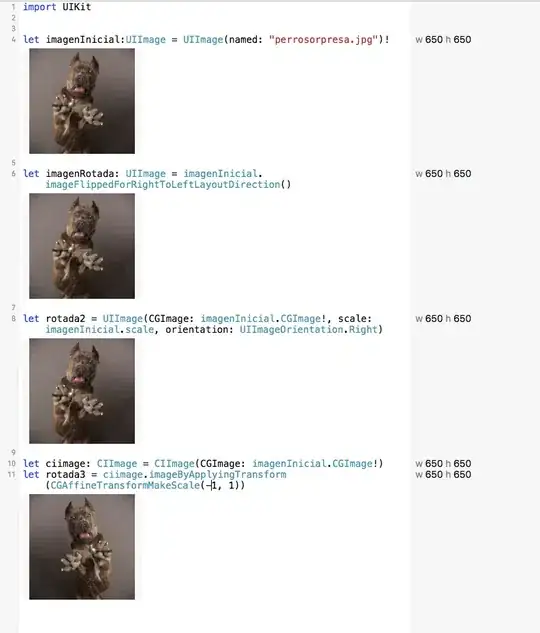
After you got the list of resolutions. You have to show it as a list or as an option to the users.
Then comes the second point.
How to make the selected resolution to play in AVPlayer.
There is an option AVPlayer has called PreferredPeakBitRate
After that, we have to set the bit rate by these values I calculated.
Apply it to the current player
open func setBitRate(_ definition: String) {
// 1. 240p = 700000
// 2. 360p = 1500000
// 3. 480p = 2000000
// 4. 720p = 4000000
// 5. 1080p = 6000000
// 6. 2k = 16000000
// 7. 4k = 45000000
var maxBitRate: Double = 0
switch definition {
case "240p":
maxBitRate = 700000
case "360p":
maxBitRate = 1500000
case "480p":
maxBitRate = 2000000
case "720p":
maxBitRate = 4000000
case "1080p":
maxBitRate = 6000000
case "2k":
maxBitRate = 16000000
case "4k":
maxBitRate = 45000000
case "Auto":
maxBitRate = 0
default:
maxBitRate = 0
}
player?.currentItem?.preferredPeakBitRate = maxBitRate
print("Playing in Bit Rate \(String(describing: player?.currentItem?.preferredPeakBitRate))")
}
Though it takes a little second by the player to update the bit rate but it will definitely work.