There are two options, here:
- As there are only two value columns, they can be plotted by separate calls to
geom_point()
. This is not recommended in general, will not produce an appropriate legend, but gives a quick answer.
- The recommended way for
ggplot2
is to reshape the value columns from wide to long format (thereby using F
and P
as id variables, so the color indicator F
isn't lost).
1. Plot data in wide format
library(ggplot2)
g <- ggplot(d, aes(factor(P), color = factor(F))) +
geom_point(aes(y = V1), shape = "triangle") +
geom_point(aes(y = V2), shape = "square")
g
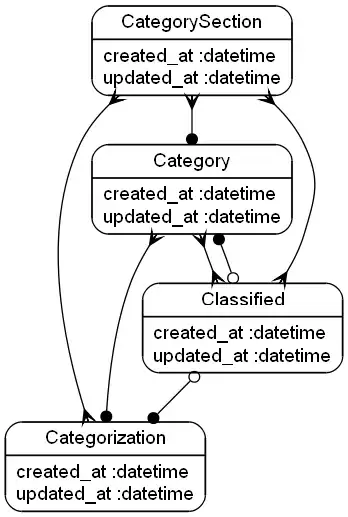
With some polishing
g +
ylab("V1, V2") +
xlab("P") +
scale_colour_manual(name = "F", values = c("red", "blue"))
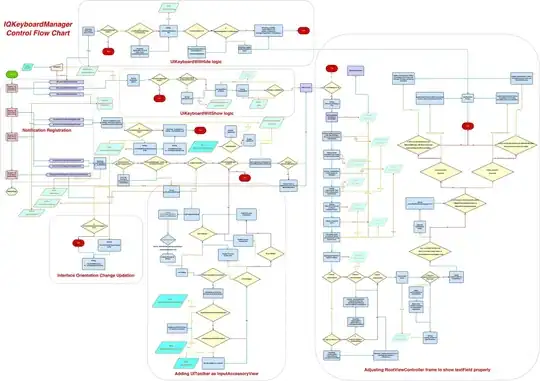
Note that both F
and P
are turned explicitely into discrete variables.
2. Plot data in long format
library(reshape2)
# reshape data from wide to long format
long <- melt(d, c("F", "P"))
g <- ggplot(long, aes(factor(P), value, shape = variable, color = factor(F))) +
geom_point()
g
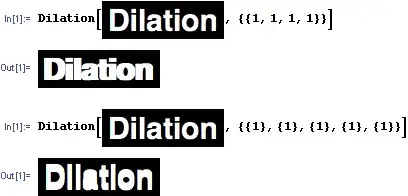
With some polishing:
g +
xlab("P") +
scale_colour_manual(name = "F", values = c("red", "blue")) +
scale_shape_manual(values = c("triangle", "square"))
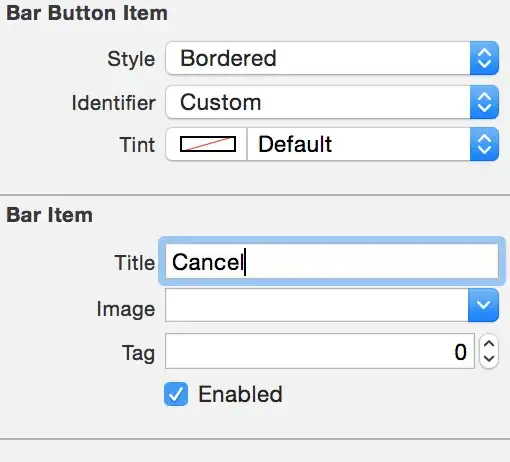
When reshaping from wide to long format it is important to specify which variables are id variables which will be repeated in every row and which are the measure variables which will constitute the values column in the long format
So,
melt(d, c("F", "P"))
and
melt(d, measure.vars = c("V1", "V2"))
produce the same result:
F P variable value
1 0 0 V1 0.5
2 0 1 V1 1.5
3 1 0 V1 0.8
4 1 1 V1 1.7
5 0 0 V2 0.7
6 0 1 V2 1.8
7 1 0 V2 0.9
8 1 1 V2 1.9
(For the sake of completeness, the data.table
version of melt()
understands pattern matching on column names, e.g., melt(d, measure.vars = patterns("V"))
.)