you said: "all I really need is to know is if there is a single swipe for that day."
Answer - aggregate query
select employeeid, TranDate, count(TranDate) as count
from History
where -- look below
Group by employeeid, TranDate
What to put in WHERE
? - depends. If you want month period then do
TranDate between '2018-10-1' and '2018-10-31 23:59:59.999'
If you want particular dates do
CAST(trandate as DATE) in ('2018-10-1', '2018-10-11', '2018-10-11')
In the latter case, you will have to build this dynamically
But in this case you're facing a new issue - what if your person never went though the door? This person will not be on the list. Then you need to letf join
this with a table that has all employees
NOW since I am not TSql super-guru but I like coding, I show you working code how it can be done programmatically. Below is a couple of snippets of code that can be combined into one
-- SETUP
create table recs (id int, dt date );
insert into recs values(1, '2018-10-1');
insert into recs values(1, '2018-10-1');
insert into recs values(2, '2018-10-2');
insert into recs values(2, '2018-10-2');
insert into recs values(2, '2018-10-3');
insert into recs values(3, '2018-10-3');
insert into recs values(3, '2018-10-3');
insert into recs values(3, '2018-10-4');
insert into recs values(3, '2018-10-1');
-- Prepare Date Grid table
DECLARE crs_dt CURSOR FOR SELECT dt FROM recs group by dt order by dt;
declare @createTbl varchar(1200) = 'create table tbl (id int, ';
declare @fetched varchar(20);
OPEN crs_dt;
FETCH NEXT FROM crs_dt into @fetched;
WHILE @@FETCH_STATUS = 0
BEGIN
set @createTbl = @createTbl + '['+@fetched+'] int'
FETCH NEXT FROM crs_dt into @fetched;
if @@FETCH_STATUS = 0
begin set @createTbl = @createTbl + ','; end
END
set @createTbl = @createTbl + ')';
CLOSE crs_dt;
DEALLOCATE crs_dt;
select @createTbl; -- just a test
execute (@createTbl)
SELECT * FROM tbl; -- just a test
-- LOAD date grid table with data
DECLARE crs_rec CURSOR FOR
SELECT id, dt, FORMAT ( dt, 'yyyy-MM-dd' ) colName,
(case count(dt) when 0 then 0 else 1 end) cnt
FROM recs group by id, dt order by dt;
declare @createInsert varchar(1200);
declare @id int, @dt date, @colName varchar(20),@yesNo int;
OPEN crs_rec;
FETCH NEXT FROM crs_rec into @id, @dt, @colName, @yesNo;
WHILE @@FETCH_STATUS = 0
BEGIN
if exists(select 1 from tbl where id = @id)
set @createInsert = 'update tbl set ['+@colName+']='+cast(@yesNo as varchar(1))+ ' where id='+ cast(@id as varchar(1000));
else
set @createInsert =
'insert into tbl (id,['+@colName+']) values ('+cast(@id as varchar(1000))+','+cast(@yesNo as varchar(1))+')';
select @createInsert; -- just a test
execute (@createInsert);
FETCH NEXT FROM crs_rec into @id, @dt, @colName, @yesNo;
END
CLOSE crs_rec;
DEALLOCATE crs_rec;
commit;
-- Lets Load data
SELECT * FROM tbl
And the result is....
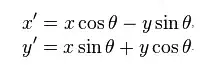
Not the fastest or most code-efficient, but certainly fun. And, 1-this is totally dynamic. 2-if you look at the result, now going back to the problem I mentioned earlier, you can join this table to full list of employees and get entire set of data, including employees who has not swipe during the selected period.