For string the Encryption and Decryption can be done using RNCryptor.
import Foundation
import RNCryptor
extension String {
func encrypt(encryptionKey: String) -> String {
let messageData = self.data(using: .utf8)!
let cipherData = RNCryptor.encrypt(data: messageData, withPassword: encryptionKey)
return cipherData.base64EncodedString()
}
func decrypt(encryptionKey: String) -> String? {
let encryptedData = Data.init(base64Encoded: self)!
if let value = try? RNCryptor.decrypt(data: encryptedData, withPassword: encryptionKey) {
let decryptedString = String(data: value, encoding: .utf8)!
return decryptedString
} else{
return nil
}
}
}
Usage:-
let encyptionKey = "password"
let unencriptedMessage = "Hello World"
print (unencriptedMessage)
let encryptedMessage = unencriptedMessage.encrypt(encryptionKey: encyptionKey)
print (encryptedMessage)
if let decryptedMessage = encryptedMessage.decrypt(encryptionKey: encyptionKey) {
print (decryptedMessage)
}
Output:-
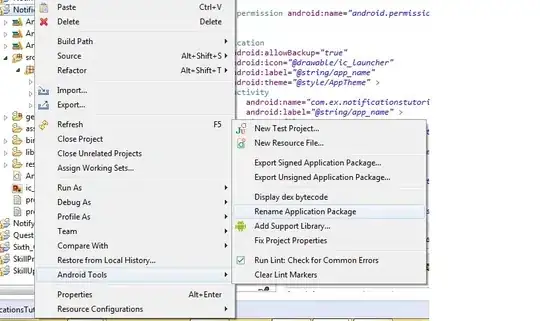
Similar Answer
Hope this Helps. Happy Coding.