int **A;
means it is a pointer to a pointer. This is often used to represent a two-dimensional array. In you program -
int *A; A = new int[10] ;
for (int j=0; j<20; j++)
A[j] = new int[20] ; // A[j] means dereferencing to the location itself. So, it should hold `int`
// as stated but not `int*` that is returned by new operator.
A
is pointer that points 10 memory locations that can hold int
. And when you say, A[j]
you are actually dereferencing to location. So, it holds an int
not an int*
. Also, there is a overflow since the number of locations A
points to 10 but not 20 memory locations.
Edit 1: OP has edited the program. It's not the same as was earlier.
Now, the program is fine, with exception of returning resources back to free store.
int **A;
A = new int*[10] ;
A
is a pointer to pointer. So, a should hold the address of a pointer or an array that can hold address of pointers. So,
A = new int*[10] ;
A
holds the beginning address of an array of pointers whose size is 10
. Now this is still wrong.
for (int j=0; j<20; j++)
A[j] = new int[20] ; // Because `A[j]` is invalid from 10-19. There is no such index where A[j] can dereference to. So, j should run from 0 to 10 instead.
With that correctected -
A[j] = new int[20] ;
Now each pointer is pointing to 20 int locations. So, there are 10*20 elements in the array.
This diagram of a 2*3 dimensional array should give you an idea. Also, you should consider @Nawaz answer of deallocating the array, since resources are managed by programmer.
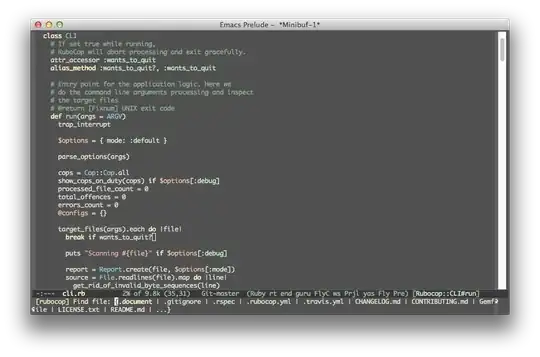