It does not seem like the library support wrapping radial chart with FlexibleXYPlot or XYPlot for that matter (see issue Responsive RadialChart "react-vis": "^1.7.7", never solved, and I couldn't get it to work), ontop of that RadialChart chart requires the width and height to be sat.
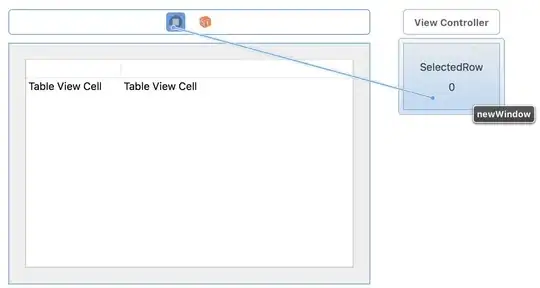
Now based on the following issues FlexibleXYPlot requires height props a pr was made to fix this issue but never merged. Based on Alex Sandiiarov implementation, the following code can be used to make the pie chart responsive:
import React, { Component } from 'react';
import ResizeObserver from 'resize-observer-polyfill';
import { RadialChart } from 'react-vis';
class App extends Component {
constructor(props) {
super(props);
this.state = {
height: 0,
width: 0
};
}
componentDidMount() {
//When mounted set our start size
this.setSize();
//Lets observe changes with resize-observer-polyfill
this.observer = new ResizeObserver(entries => this.setSize());
//Lets observe our node
this.observer.observe(this.node);
}
componentWillUnmount() {
this.observer.disconnect();
}
setSize = () => {
const { height, width } = this.node.getBoundingClientRect();
this.setState({ height, width });
};
render() {
const { height, width } = this.state;
return (
<div
ref={node => {
if (node) {
this.node = node;
}
}}
style={{ width: '100vw', height: '100vh' }}
>
<RadialChart
className={'donut-chart-example'}
getAngle={d => d.theta}
data={[
{ theta: 2, className: 'custom-class' },
{ theta: 6 },
{ theta: 2 },
{ theta: 3 },
{ theta: 1 }
]}
width={width}
height={height}
padAngle={0.04}
colorType="literal"
/>
</div>
);
}
}
export default App;
Hope this helps you or points you in a useful direction :)