You can write a function that takes the data frame and Place
as inputs then loop through all the values in Place
column to create the corresponding plots.
library(tidyverse)
df <- data_frame(
Place = c(rep(c("A", "B", "C"), each = 3)),
Num_of_Visits = seq(1:9),
Day = rep(c("Sunday", "Monday", "Tuesday"), 3)
)
df <- df %>%
mutate(Day = factor(Day, levels = c("Sunday", "Monday", "Tuesday")))
my_plot <- function(df, myvar) {
ggplot(data = df %>% filter(Place == myvar),
aes(x = Day, y = Num_of_Visits, group = 1)) +
geom_line(color = "#00AFBB", size = 0.5) +
theme(axis.text.x = element_text(angle = 90, vjust = 0.5))
}
# test
my_plot(df, 'A')

Loop through Place
var, create plots & store them in a list using purrr::map()
plot_list <- unique(df$Place) %>%
purrr::set_names() %>%
purrr::map( ~ my_plot(df, .x))
str(plot_list, max.level = 1)
#> List of 3
#> $ A:List of 9
#> ..- attr(*, "class")= chr [1:2] "gg" "ggplot"
#> $ B:List of 9
#> ..- attr(*, "class")= chr [1:2] "gg" "ggplot"
#> $ C:List of 9
#> ..- attr(*, "class")= chr [1:2] "gg" "ggplot"
Display all plots with purrr::walk()
purrr::walk(plot_list, print)
Save all plots to PNG files using purrr::iwalk()
purrr::iwalk(plot_list,
~ ggsave(plot = .x,
filename = paste0("Plot_", .y, ".png"),
type = 'cairo', width = 6, height = 6, dpi = 150)
)
Combine all plot together if needed using cowplot::plot_grid()
library(cowplot)
do.call(plot_grid, c(plot_list,
align = "h",
axis = 'tb',
ncol = 3))
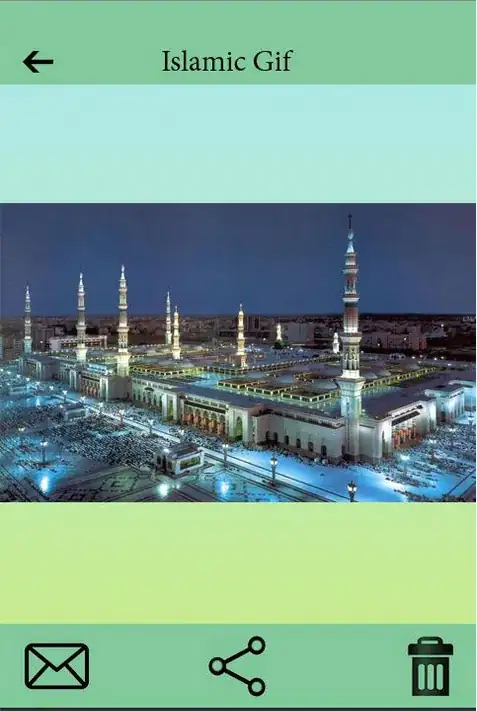
Created on 2018-10-19 by the reprex package (v0.2.1.9000)