Mmmm... your result images are identical to your foreground images because although the foreground images have an alpha/transparency layer, they are fully opaque and completely conceal your backgrounds. You need to have a rethink!
You can use ImageMagick in the Terminal to inspect your images. So, let's look at your foreground image:
identify -verbose fg.png
Sample Output
Image: fg.png
Format: PNG (Portable Network Graphics)
Mime type: image/png
Class: DirectClass
Geometry: 118x128+0+0
Units: Undefined
Colorspace: sRGB
Type: PaletteAlpha <--- Image does have alpha/transparency layer
Base type: Undefined
Endianess: Undefined
Depth: 8-bit
Channel depth:
Red: 8-bit
Green: 8-bit
Blue: 8-bit
Alpha: 1-bit
Channel statistics:
Pixels: 15104
Red:
min: 30 (0.117647)
...
...
Alpha:
min: 255 (1) <--- ... but alpha layer is fully opaque
max: 255 (1)
mean: 255 (1)
standard deviation: 0 (0)
kurtosis: 8.192e+51
skewness: 1e+36
entropy: 0
So there is no point pasting a fully opaque image over a background as it will fully conceal it.
If we punch a transparent hole in your foreground image with ImageMagick:
convert fg.png -region 100x100+9+14 -alpha transparent fg.png
It now looks like this:
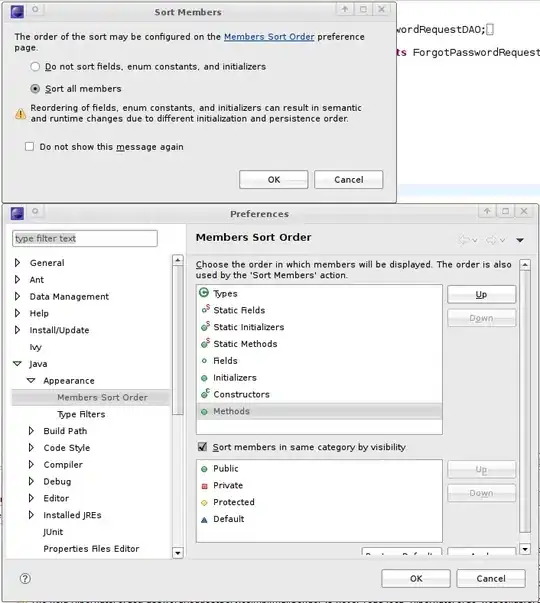
And if we then run your code:
#!/usr/local/bin/python3
from PIL import Image
background = Image.open('bg.png')
foreground = Image.open('fg.png')
background.paste(foreground, (0, 0), foreground)
background.save('result.png')
It works:
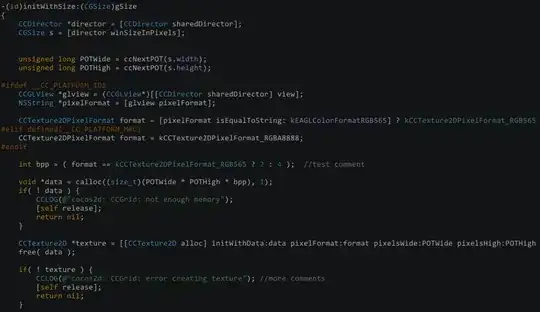
So the moral of the story is that your foreground image either needs some transparency to allow the background to show through, or you need to use some blending mode to choose one or the other of the foreground and background images at each location, or to choose some combination - e.g. the average of the two, or the brighter of the two.
If you want to average the two images, or in fact, do any other blending mode, you could consider using Pillow's ImageChops
module - documentation here. So, an average would look like this:
#!/usr/local/bin/python3
from PIL import Image, ImageChops
bg = Image.open('bg.png')
fg = Image.open('fg.png')
# Average the two images, i.e. add and divide by 2
result = ImageChops.add(bg, fg, scale=2.0)
result.save('result.png')
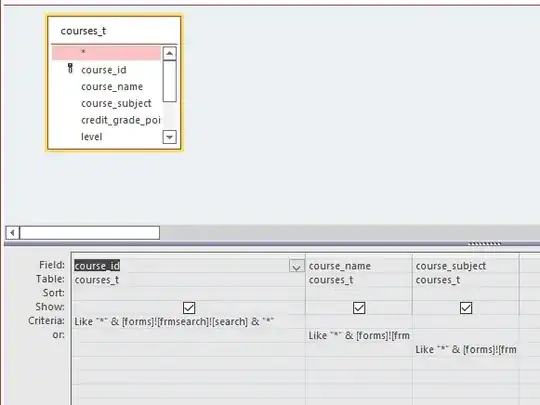