if I understand your question the right way, a DelegatingHandler is what you need.
It could be like this:
public class RequestHandler : DelegatingHandler
{
protected override Task<HttpResponseMessage> SendAsync(HttpRequestMessage request, CancellationToken cancellationToken)
{
Console.WriteLine(request.RequestUri);
request.RequestUri = new Uri(toEnglishNumber(request.RequestUri.ToString()));
Console.WriteLine(request.RequestUri);
return base.SendAsync(request, cancellationToken);
}
public static string toEnglishNumber(string input)
{
string[] persian = new string[10] { "۰", "۱", "۲", "۳", "۴", "۵", "۶", "۷", "۸", "۹" };
for (int j = 0; j < persian.Length; j++)
input = input.Replace(persian[j], j.ToString());
return input;
}
}
Every request runs first in the handler where you can do your staff.
You need to register it globally:
public static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
config.MessageHandlers.Add(new RequestHandler());
// Web API routes
config.MapHttpAttributeRoutes();
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "api/{controller}/{id}",
defaults: new { id = RouteParameter.Optional }
);
}
}
And for a simple test a controller:
public class TestController : ApiController
{
public IHttpActionResult Get(int number)
{
return this.Ok($"Hello World {number}.");
}
}
And it is possible to do this:
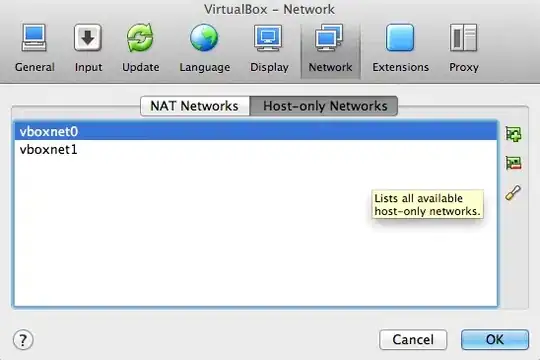
As further reference, see the web api poster: https://www.asp.net/media/4071077/aspnet-web-api-poster.pdf
Happy coding!