using only features available in .net 2.0
Assuming you have installed the multi-target packages for Visual Studio, you should be able to target a specific framework when you create a project. You can also change the targeted framework via the project's property pages.
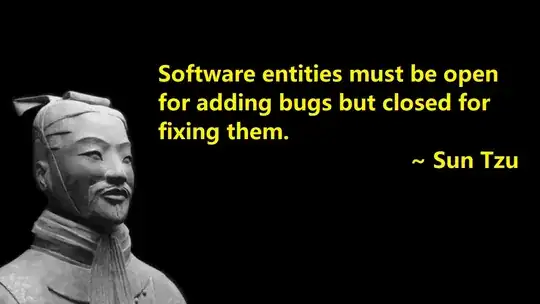
If you also need to specify the supported language version, this can be set via the project properties Build Tab by clicking on the "Advanced" button. In this case, ISO-2 is selected to correspond to C# V2 that was released as part of VS2005 along with .Net 2.0.
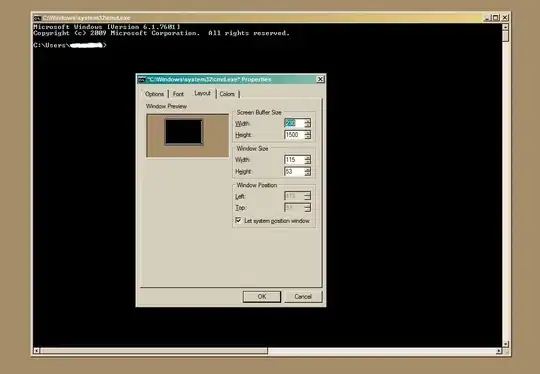
If you need information on multi-targeting, see:
With the project environment thus configured, you will only be able to use features available in .Net 2.0 and C# 2.0. This includes what you will see in the Object Browser.
Now to the issue at hand, sorting the Dictionary<string, float>
contents by Value.
A simple way is to extract out the key-value pairs into a class supports sorting. The example below uses a List<KeyValuePair<string, float>>
to hold the values. The list's sort method is passed a System.Comparison delegate to the that method defines the comparison logic. The comparison logic is designed to sort by both value (descending) and secondarily on key (ascending) if the values are equal.
public void Test()
{
Dictionary<string, float> dict = new Dictionary<string, float>();
dict.Add("A1", 3);
dict.Add("A2", 30);
dict.Add("Z", 30);
dict.Add("A3", 30);
dict.Add("F", 5);
List<KeyValuePair<string, float>> sorted = new List<KeyValuePair<string, float>>(dict);
sorted.Sort(new Comparison<KeyValuePair<string, float>>(CompareKvp));
foreach(KeyValuePair<string, float> kvp in sorted)
{
Console.WriteLine(kvp.ToString());
}
}
private static Int32 CompareKvp(KeyValuePair<string, float> kvp1, KeyValuePair<string, float> kvp2)
{
int ret = - kvp1.Value.CompareTo(kvp2.Value); // negate for descending
if (ret == 0)
{
ret = kvp1.Key.CompareTo(kvp2.Key); // Ascend comp for Key
}
return ret;
}
This example will yield ordered key-value pairs:
[A2, 30]
[A3, 30]
[Z, 30]
[F, 5]
[A1, 3]