You can do it with numpy
like this. I used this image for testing:
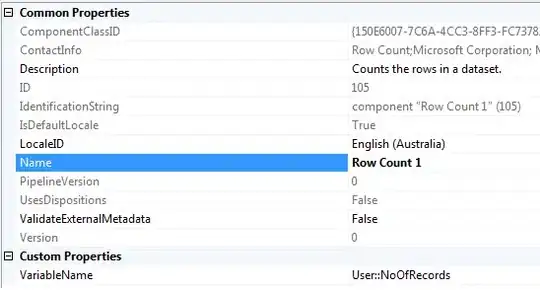
The code is:
#!/usr/bin/env python3
import numpy as np
from PIL import Image
# Load image and make into NumPy array
im=np.array(Image.open('image.png').convert('RGB'),dtype=np.uint8)
# Colour we are looking for
red=np.array([255,0,0],dtype=np.uint8)
# Find the red pixels
np.where(np.all(im==red,axis=-1))
The result is:
(array([10, 10, 10, 11, 11, 11]), array([10, 11, 12, 10, 11, 12]))
which means the first red pixel is at 10,10, the second at 10,11 etc.
On my Mac, that takes 7.03ms for an 800x600 image.
Just to check, we can dump the image using ImageMagick like this and check where the red pixels are:
convert image.png txt:
Output
# ImageMagick pixel enumeration: 23,22,65535,srgb
0,0: (0,65535,0) #00FF00 lime
1,0: (0,65535,0) #00FF00 lime
2,0: (0,65535,0) #00FF00 lime
...
...
7,10: (0,65535,0) #00FF00 lime
8,10: (0,65535,0) #00FF00 lime
9,10: (0,65535,0) #00FF00 lime
10,10: (65535,0,0) #FF0000 red <--- red
11,10: (65535,0,0) #FF0000 red <--- red
12,10: (65535,0,0) #FF0000 red <--- red
13,10: (0,65535,0) #00FF00 lime
14,10: (0,65535,0) #00FF00 lime
...
...
8,11: (0,65535,0) #00FF00 lime
9,11: (0,65535,0) #00FF00 lime
10,11: (65535,0,0) #FF0000 red <--- red
11,11: (65535,0,0) #FF0000 red <--- red
12,11: (65535,0,0) #FF0000 red <--- red
13,11: (0,65535,0) #00FF00 lime
14,11: (0,65535,0) #00FF00 lime
15,11: (0,65535,0) #00FF00 lime
...
...
If the red pixels are often near the top of the image, you could do 1/4 of the image at a time, or if you have 4 CPU cores, you could do 1/4 of the image on each core:
np.where(np.all(im[0:height//4,:,:]==red,axis=-1))
That takes 1.7ms instead of 7ms.