Hy I am new to php and trying to destroy a session according to the php documentation here: http://php.net/manual/en/function.session-destroy.php so I am using this code:
<?php
session_start();
echo 'cokkies before delete session';
var_dump($_COOKIE);
var_dump($_SESSION);
echo '-------------- <br>';
$_SESSION = array();
if (ini_get("session.use_cookies")) {
$params = session_get_cookie_params();
setcookie(session_name(), '', time() - 42000,
$params["path"], $params["domain"],
$params["secure"], $params["httponly"]
);
}
session_destroy();
echo 'cokkies after delete session';
var_dump($_COOKIE);
var_dump($_SESSION);
?>
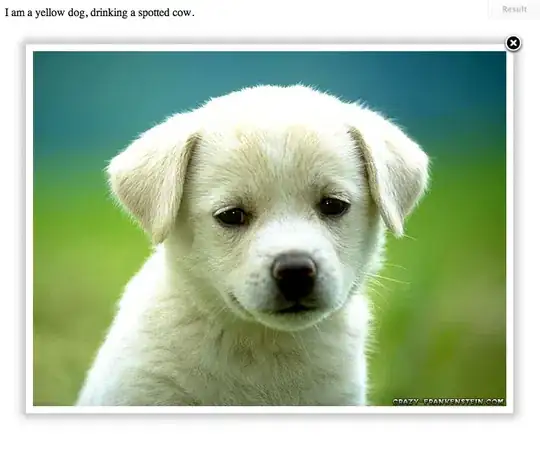
So is then the session id on the server destroyed at all and just the id in the cookie stays alive? And overall why does it work out like this. Thanks for the answers